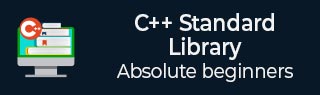
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Map Library - operator[] Function
Description
The C++ function std::map::operator[] if key k matches an element in the container, then method returns a reference to the element.
Declaration
Following is the declaration for std::map::operator[] function form std::map header.
C++98
mapped_type& operator[] (const key_type& k);
C++11
mapped_type& operator[] (const key_type& k);
Parameters
k − Key of the element whose mapped value is accessed.
Return value
Returns a reference to the element associated with key k.
Exceptions
This member doesn't throw exception.
Time complexity
Logarithmic i.e. O(lon n)
Example
The following example shows the usage of std::map::operator[] function.
#include <iostream> #include <map> using namespace std; int main(void) { /* Initializer_list constructor */ map<char, int> m = { {'a', 1}, {'b', 2}, {'c', 3}, {'d', 4}, {'e', 5}, }; cout << "Map contains following elements" << endl; cout << "m['a'] = " << move(m['a']) << endl; cout << "m['b'] = " << move(m['b']) << endl; cout << "m['c'] = " << move(m['c']) << endl; cout << "m['d'] = " << move(m['d']) << endl; cout << "m['e'] = " << move(m['e']) << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
Map contains following elements m['a'] = 1 m['b'] = 2 m['c'] = 3 m['d'] = 4 m['e'] = 5
map.htm
Advertisements