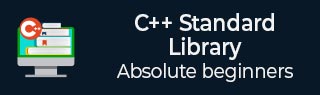
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Map Library - insert() Function
Description
The C++ function std::map::insert() extends map by inserting new element from initializer list. This function increases container size by one.
Declaration
Following is the declaration for std::map::insert() function form std::map header.
C++11
void insert (initializer_list<value_type> il);
Parameters
il − Initializer list.
Return value
None.
Exceptions
This member function doesn't throw exception.
Time complexity
Logarithmic i.e. O(log n).
Example
The following example shows the usage of std::map::insert() function.
#include <iostream> #include <map> using namespace std; int main(void) { map<char, int> m = { {'b', 2}, {'c', 3}, {'d', 4}, }; m.insert({{'e', 5}, {'a', 1}}); cout << "Map contains following elements" << endl; for (auto it = m.begin(); it != m.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
Map contains following elements a = 1 b = 2 c = 3 d = 4 e = 5
map.htm
Advertisements