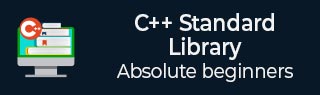
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ List::rend() Function
The C++ std::list::rend() function is used to retrieve a reverse iterator to the element following the last element of the reversed list.
This function corresponds to the element preceding the first element of the non-reversed list. The elements of this list act as a placeholder, attempting to access it results in undefined behavior. In C++, iterators are used to point at the memory addresses of STL(standard template library) containers.
The rend() function is similar to the crend() function, where the crend() function returns a constant reverse iterator to the element following the last element of the reversed list, whereas the rend() function returns a reverse iterator following to the last element of the reversed list.
Syntax
Following is the syntax of the C++ std::list::rend() function −
const_reverse_iterator rend();
Parameters
- It does not accept any parameter.
Return Value
This function returns a reverse iterator to the element following the last element of the reversed list.
Example 1
In the following program, we are using the C++ std::list::rend() function to retrieve a reverse iterator to the element following the last element of the current list {10, 20, 30, 40}.
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {10, 20, 30, 40}; cout<<"List elements are: "; for(int n : num_list) { cout<<n<<" "; } auto it = num_list.rend(); cout<<"\nA reverse iterator of the last element: "; cout<<*it; }
Output
Following is the output of the above program −
List elements are: 10 20 30 40 A constant reverse iterator of the last element: 4
Example 2
Apart from the int-type list element, you can also retrieve an iterator of any other type list element like char and string list content.
Following is another example of the C++ std::list::rend() function. Here, we are creating a list(type char) named symbols with the values {'@', '#', '$', '%', '&', '*'}. Then using the rend() function, we are trying to retrieve a reverse iterator following to the last element of the reversed list.
#include<iostream> #include<list> using namespace std; int main() { //create a list list<char> symbols = {'@', '#', '$', '%', '&', '*'}; cout<<"List elements are: "; for(char v : symbols) { cout<<v<<" "; } auto it = symbols.rend(); cout<<"\nA reverse iterator of the last element: "; cout<<*it; }
Output
This will generate the following output −
List elements are: @ # $ % & * A reverse iterator of the last element:
Example 3
If the list type is a string.
In this example, we are creating a list(type string) named names with the values {"Mukesh", "Rahul", "Mohan", "Shavita"}. Then, using the rend() function, we are trying to retrieve a reverse iterator following to the last element of the reversed list.
#include<iostream> #include<list> using namespace std; int main() { //create a list list<string> names = {"Mukesh", "Rahul", "Mohan", "Shavita"}; cout<<"List elements are: "; for(string s : names ) { cout<<s<<" "; } auto it = names.rend(); cout<<"\nA reverse iterator of the last element: "; cout<<*it; }
Output
The above program produces the following output −
List elements are: Mukesh Rahul Mohan Shavita A reverse iterator of the last element: Segmentation fault
Example 4
Using for loop along with the rend() function to retrieve a reverse iterator of the reversed list.
In this example, we use the rend() function along with the for loop to loop through the current container {1, 2, 3, 4 ,5, 6, 7, 8, 9, 10} and retrieve a reverse iterator of all elements of the reversed list.
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {1, 2, 3, 4 ,5, 6, 7, 8, 9, 10} ; cout<<"List elements are: "; for(int n : num_list) { cout<<n<<" "; } cout<<"\nThe reverse iterator of an elements are : "; for (auto it = num_list.crbegin(); it != num_list.rend(); it++) { cout<<*it<<" "; } }
Output
On executing the above program, it will produce the following output −
List elements are: 1 2 3 4 5 6 7 8 9 10 The reverse iterator of an elements are : 10 9 8 7 6 5 4 3 2 1