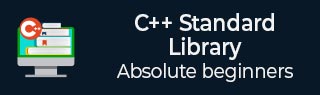
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ List::remove_if() Function
The C++ std::list::remove_if() function is used to remove an element from the list that fulfills the condition. It accepts single parameters as pred and removes all the elements from the current list which fulfill the condition. A pred is nothing but, is a predicate function, it is a user-defined function and accepts only one parameter, which must match the type of items kept in the List.
The remove_if() function does not return any value because the function return type is void and removes all elements in the list for which the predicate function returns true.
Syntax
Following is the syntax of the C++ std::list::remove_if() function −
void remove_if (Predicate pred);
Parameters
pred − It is a unary predicate function that takes a value of the same type and returns true for those values to be removed from the list.
Return Value
This function does not return any value.
Example 1
In the following program, we define a predicate function named Con(int n) that accepts an int-type parameter, and we use this function to filter out all values greater than 2. Then, we pass this function as a parameter to the remove_if() function to remove all list elements that are greater than 2.
#include<iostream> #include<list> using namespace std; //custom function to check condition bool Con(int n) { return (n>2); } int main(void) { list<int> num_list = {1, 2, 3, 4, 5}; cout<<"List elements before remove_if operation: "<<endl; for(int n : num_list) { cout<<n<<" "; } //using remove_if() function num_list.remove_if(Con); cout<<"\nList elements after remove_if operation: "; for(int n : num_list) { cout<<n<<" "; } return 0; }
Output
This will generate the following output −
List elements before remove_if operation: 1 2 3 4 5 List elements after remove_if operation: 1 2
Example 2
Following is another example of the C++ std::list::remove_if() function. Here, we define a predicate function named filter_length(string len) that accepts a string as a parameter. Then, we pass this function as a parameter to the remove_if() function to remove all the string elements in the list whose length is greater than 3.
#include<iostream> #include<list> using namespace std; bool filter_length(string n) { return (n.length()>3); } int main(void) { list<string> num_list = {"Hello", "how", "are", "you"}; cout<<"List elements before remove_if operation: "<<endl; for(string n : num_list) { cout<<n<<" "; } //using remove_if() function num_list.remove_if(filter_length); cout<<"\nList elements after remove_if operation: "; for(string n : num_list) { cout<<n<<" "; } return 0; }
Output
Following is the output of the above program −
List elements before remove_if operation: Hello how are you List elements after remove_if operation: how are you
Example 3
Remove even numbers from the list.
In this example, we define a predicate function named filter_evens(int n) that accepts an int-type parameter. Then, we pass this function as a parameter to the remove_if() function to remove all the even elements (or numbers) from the current list {1,2,3,4,5,6,7,8,9,10}.
#include<iostream> #include<list> using namespace std; //custom function to check condition bool filter_evens(int n) { return (n%2 == 0); } int main(void) { list<int> num_list = {1,2,3,4,5,6,7,8,9,10}; cout<<"List elements before remove_if operation: "<<endl; for(int n : num_list) { cout<<n<<" "; } //using remove_if() function num_list.remove_if(filter_evens); cout<<"\nAfter removing even numbers from list elements are: "; for(int n : num_list) { cout<<n<<" "; } return 0; }
Output
On executing the above program, it produces the following output −
List elements before remove_if operation: 1 2 3 4 5 6 7 8 9 10 After removing even numbers from list elements are: 1 3 5 7 9