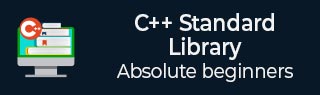
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ List::empty() Function
The C++ std::list::empty() function is used to checks whether the list is empty or not.
It returns a boolean value true if and only if the current list is empty; else returns false. In C++, the bool data type is treated the same as the boolean data type in other programming languages, which can take the value true(1) or false(0). It returns 1 for true and 0 for false conditions in the result.
Syntax
Following is the syntax of the C++ std::list::empty() function −
bool empty() const;
Parameters
- It does not accept any parameter.
Return Value
This function returns true if list is empty; false otherwise.
Example 1
If the current list is not empty, the empty() function returns 0.
In the following program, we are using the C++ std::list::empty() function to verify whether the current list {1, 2,3 4, 5} is empty or not.
#include<iostream> #include<list> using namespace std; int main(void) { //create an integer list list<int> lst = {1, 2, 3, 4, 5}; cout<<"The list elements are: "; for(int l: lst){ cout<<l<<" "; } cout<<"\nIs list is empty or not? "<<lst.empty(); }
Output
Following is the output of the above program −
The list elements are: 1 2 3 4 5 Is list is empty or not? 0
Example 2
If the current list is empty, the empty() function returns 1.
Following is another example of the C++ std::list::empty() function, here we are creating an empty list(type char), and using the empty() function, we are trying to verify whether this list is empty or not.
#include<iostream> #include<list> using namespace std; int main(void) { list<char> lst = {}; cout<<"The list elements are: "; for(char l: lst){ cout<<l<<" "; } cout<<"\nThe list size is: "<<lst.size(); cout<<"\nIs list is empty or not? "<<lst.empty(); }
Output
The above program produces the following output −
The list elements are: The list size is: 0 Is list is empty or not? 1
Example 3
Using conditional statements to check whether the list is empty or not.
In the following example, we are using the empty() function result in the conditional statement to check whether the current list named cities {"Delhi", "Hyderabad", "Lucknow", "Prayagraj"} is empty or not.
#include<iostream> #include<list> using namespace std; int main(void) { list<string> cities = {"Delhi", "Hyderabad", "Lucknow", "Prayagraj"}; cout<<"The list elements are: "; for(string l: cities){ cout<<l<<" "; } cout<<"\nThe list size is: "<<cities.size(); int res = cities.empty(); if(res==1){ cout<<"\nList is an empty list."; } else{ cout<<"\nList is not empty."; } }
Output
On executing the above program, it produces the following output −
The list elements are: Delhi Hyderabad Lucknow Prayagraj The list size is: 4 List is not empty.
Example 4
In this example, we are creating a list named marks with the values {80,90,70,95,88,78}. Using the clear() function, we clear the list by removing all the elements, and then we use the empty() function result in the conditional statement to verify whether the list is empty or not.
#include<iostream> #include<list> using namespace std; int main(void) { list<int> marks = {80,90,70,95,88,78}; cout<<"The list elements are: "; for(int l: marks){ cout<<l<<" "; } cout<<"\nThe list size is: "<<marks.size(); //clear the list marks.clear(); cout<<"\nAfter clear the list size is: "<<marks.size(); int res = marks.empty(); if(res==1){ cout<<"\nList is an empty list."; } else{ cout<<"\nList is not empty."; } }
Output
After executing the above program, it generates the following output −
The list elements are: 80 90 70 95 88 78 The list size is: 6 After clear the list size is: 0 List is an empty list.