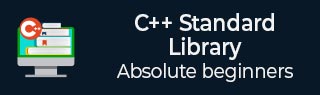
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ List::emplace() Function
The C++ std::list::emplace() function is used to insert a new element into the container directly before the pos(where the pos is an iterator before which the new element will be constructed).
Using an inbuilt function begin() and end(), you can specify the beginning and end position of an element in the container and insert the element using emplace() function. It extends the list size by one after inserting a new element into the list. It throws an error if we give the position value like (0,1,2,3..n).
Syntax
Following is the syntax of the C++ std::list::emplace() function −
iterator emplace (const_iterator position, val);
Parameters
- pos − It is the position in the list where the new element is to be inserted.
- val − It specifies the new element value is to be inserted in the list.
Return Value
This function returns a random access iterator which points to the newly emplaced element.
Example 1
Insert a new element at the end(or at the last position) of the list.
In the following program, we are using the C++ std::list::emplace() function to insert a new element value 40 in the current list {10, 20, 30 } at the specified position(at the end).
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {10, 20, 30}; cout<<"List elements before emplace operation: "; for(int n : num_list) { cout<<n<<" "; } //using emplace() function num_list.emplace(num_list.end(), 40); cout<<"\nList elements after emplace operation: "; for(int n : num_list) { cout<<n<<" "; } return 0; }
Output
This will generate the following output −
List elements before emplace operation: 10 20 30 List elements after emplace operation: 10 20 30 40
Example 2
Insert a new element at the beginning(or at the first position) of the list.
Following is another example of the C++ std::list::emplace() function. Here, we are creating a list(type char) named symbols with the values {'@', '#', '$', '%'}. Then using the emplace() function, we are trying to insert a new char element '&' at the specified position(at the beginning) in this list.
#include<iostream> #include<list> using namespace std; int main() { //create a list list<char> symbols = {'@', '#', '$', '%'}; cout<<"List elements before emplace operation: "; for(char n : symbols) { cout<<n<<" "; } //using emplace() function symbols.emplace(symbols.begin(), '&'); cout<<"\nList elements after emplace operation: "; for(char n : symbols) { cout<<n<<" "; } return 0; }
Output
Following is the output of the above program −
List elements before emplace operation: @ # $ % List elements after emplace operation: & @ # $ %
Example 3
Insert an element in an empty list.
In this example, we are creating a list(type string) named cities with the values {"Delhi", "Mumbai", "Hyderabad", "Lucknow"}. Then, using the emplace() function, we are trying to insert the string value "Prayagraj" in this list at the specified position(at the end).
#include<iostream> #include<list> using namespace std; int main() { //create a list list<string> cities = {"Delhi", "Mumbai", "Hyderabad", "Lucknow"}; cout<<"List elements before emplace operation: "; for(string c : cities) { cout<<c<<" "; } //using emplace() function cities.emplace(cities.end(), "Prayagraj"); cout<<"\nList elements after emplace operation: "; for(string c : cities) { cout<<c<<" "; } return 0; }
Output
On executing the above program, it will produce the following output −
List elements before emplace operation: Delhi Mumbai Hyderabad Lucknow List elements after emplace operation: Delhi Mumbai Hyderabad Lucknow Prayagraj
Example 4
Insert elements dynamically in the list.
In the following program, we are creating a list(type int) with the values {1,2,3}. Then using the emplace() function inside the for loop to insert an elements dynamically in the current list at the specified position(at the end).
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {1,2,3}; cout<<"List elements before emplace operation: "; for(int n : num_list) { cout<<n<<" "; } //using for loop for(int i = 3; i<10; i++) { num_list.emplace(num_list.end(), i+1); } cout<<"\nList elements after emplace operation: "; for(int l : num_list) { cout<<l<<" "; } return 0; }
Output
The above program generate the following output −
List elements before emplace operation: 1 2 3 List elements after emplace operation: 1 2 3 4 5 6 7 8 9 10