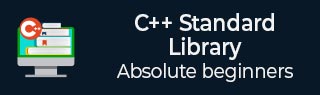
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ List::begin() Function
The C++ std::list::begin() function is used to retrieve an iterator to the first element of the list.
In C++, iterators are used to point at the memory addresses of STL(standard template library) containers. The begin() function is different from the front() function, but it is similar to the cbegin() function in C++ std::list. If the list is empty, the returned iterator will be equal to end().
The begin() function returns the bidirectional iterator, whereas the front() function returns the reference of the first element of the list.
Syntax
Following is the syntax of the C++ std::list::begin() function −
iterator begin();
Parameters
- It does not accepts any parameter.
Return Value
This function returns an iterator to the first element of the list container.
Example 1
In the following program, we are using the C++ std::list::begin() function to retrieve an iterator to the first ( or beginning) element of the current list {10, 20, 30, 40, 50}.
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {10, 20, 30, 40, 50}; cout<<"List elements are: "; for(int n : num_list) { cout<<n<<" "; } auto ri = num_list.begin(); cout<<"\nThe iterator of the first element: "; cout<<*ri; }
Output
Following is the output of the above program −
List elements are: 10 20 30 40 50 The iterator of the first element: 10
Example 2
Apart from the int-type list element, you can also retrieve an iterator of any other type list element like char and string list content.
Following is another example of the C++ std::list::begin() function. Here, we are creating a list(type char) named vowels with the values {'a', 'e', 'i', 'o', 'u'}. Then using the begin() function, we are trying to retrieve an iterator to the first element of the current list.
#include<iostream> #include<list> using namespace std; int main() { //create a list list<char> vowels = {'a', 'e', 'i', 'o', 'u'}; cout<<"List elements are: "; for(char v : vowels) { cout<<v<<" "; } auto ri = vowels.begin(); cout<<"\nThe iterator of the first element: "; cout<<*ri; }
Output
This will generate the following output −
List elements are: a e i o u The iterator of the first element: a
Example 3
If the list type is a string.
In this example, we are creating a list(type string) named fname with the values {"Rohan", "Reema", "Geeta", "Seema"}. Then, using the begin() function, we are trying to retrieve an iterator to the first element of the current list.
#include<iostream> #include<list> using namespace std; int main() { //create a list list<string> names = {"Rohan", "Reema", "Geeta", "Seema"}; cout<<"List elements are: "; for(string s : names) { cout<<s<<" "; } auto ri = names.begin(); cout<<"\nThe iterator of last element: "; cout<<*ri; }
Output
The above program produces the following output −
List elements are: Rohan Reema Geeta Seema The iterator of last element: Rohan
Example 4
Using for loop along with the begin() function to retrieve the iterator of the container element.
In this example, we use the begin() function along with the for loop to loop through the current container {1, 2, 3, ,4 ,5} and retrieve an iterator of all elements in the container starting from the first element.
#include<iostream> #include<list> using namespace std; int main() { //create a list list<int> num_list = {1, 2, 3, 4 ,5}; cout<<"List elements are: "; for(int n : num_list) { cout<<n<<" "; } cout<<"\nA reverse iterator of an elements are : "; for(auto i = num_list.begin(); i!=num_list.end(); i++) { cout<<*i<<" "; } }
Output
On executing the above program, it will produce the following output −
List elements are: 1 2 3 4 5 A reverse iterator of an elements are : 1 2 3 4 5