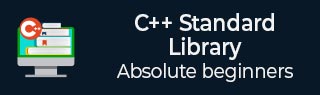
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ iterator::begin() Function
The C++ iterator::begin() function returns a pointer pointing to the container's first element. This pointer is bidirectional since it can point in any direction of the container. This function is available in the std namespaces map associative container class template, where key-value pairs are used to store the elements.
Iterators operate as a bridge that connects algorithms to STL containers and allows the modification of the data present inside the container. To get the desired outcome, you can use them to iterate over the container, access and assign the values, and run different operators over them.
Syntax
Following is the syntax for C++ iterator::begin() Function −
auto begin(Container& cont) ` -> decltype(cont.begin()); auto begin(const Container& cont) ` -> decltype(cont.begin()); Ty *begin(Ty (& array)[Size]);
Parameters
- cont − it indicates the container that returns cont.begin().
- array − an array of objects of type Ty.
Example 1
Let's consider the following example, where we are going to use the begin() function and retrive the output.
#include<iostream> #include<iterator> #include<vector> using namespace std; int main() { vector<int> array = {2,3,4,12,14}; vector<int>::iterator ptr; cout << "The Elements are : "; for (ptr = array.begin(); ptr < array.end(); ptr++) cout << *ptr << " "; return 0; }
Output
When we compile and run the above program, this will produce the following result −
The Elements are : 2 3 4 12 14
Example 2
Consider the following example, where we are going to use map with the begin() function and retrieving the output.
#include <iostream> #include <iterator> #include <map> using namespace std; int main() { map<string,int> Student = { {"Amar", 20}, {"Akbar", 21}, { "Antony" ,32}, }; map<string,int>::const_iterator iterator; iterator = Student.begin(); cout << "Name"<<"\t" <<"Age" << "\n"; while (iterator != Student.end() ) { cout << iterator->first <<"\t" << iterator->second << "\n"; ++iterator; } cout << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Name Age Akbar 21 Amar 20 Antony 32
Example 3
Look into the another scenario, where we are going to use begin() function and retrieving the output.
#include <iostream> #include <vector> int main () { int foo[] = {1,2,3,4,5}; std::vector<int> bar; for (auto it = std::begin(foo); it!=std::end(foo); ++it) bar.push_back(*it); std::cout << "bar contains:"; for (auto it = std::begin(bar); it!=std::end(bar); ++it) std::cout << ' ' << *it; std::cout << '\n'; return 0; }
Output
On running the above program, it will produce the following result −
bar contains: 1 2 3 4 5