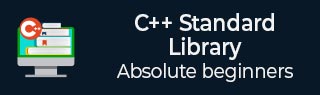
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ iomanip Library - get_time Function
Description
This function accesses the input sequence by first constructing an object of type basic_istream::sentry. Then (if evaluating the sentry object is true), it calls time_get::get (using the stream's selected locale) to perform both the extraction and the parsing operations, and adjusts the stream's internal state flags accordingly. Finally, it destroys the sentry object before returning.
It is used to extracts characters from the input stream it is applied to, and interprets them as time and date information as specified in argument fmt. The obtained data is stored at the struct tm object pointed by tmb.
Declaration
Following is the declaration for std::get_time function.
template <class charT> /*unspecified*/ get_time (struct tm* tmb, const charT* fmt);
Parameters
tmb − Pointer to an object of type struct tm where the time and date information extracted is stored. struct tm is a class defined in header <ctime>.
fmt − C-string used by time_get::get as format string (see time_get::get). charT is the character type in the c-string.
Return Value
Unspecified. This function should only be used as a stream manipulator.
Errors are signaled by modifying the stream's internal state flags −
flag | error |
---|---|
eofbit | The input sequence has no more characters available (end-of-file reached). |
failbit | Either no characters were extracted, or the characters extracted could not be interpreted as a valid monetary value. |
badbit | Error on stream (such as when this function catches an exception thrown by an internal operation).When set, the integrity of the stream may have been affected. |
Exceptions
Basic guarantee − if an exception is thrown, the object is in a valid state.
It throws an exception of member type failure if the resulting error state flag is not goodbit and member exceptions was set to throw for that state.
Any exception thrown by an internal operation is caught and handled by the function, setting badbit. If badbit was set on the last call to exceptions, the function rethrows the caught exception.
Data races
Accesses the array pointed by fmt Modifies the object pointed by tmb and the stream object from which it is extracted.
Concurrent access to the same stream object may cause data races, except for the standard stream objects cin and wcin when these are synchronized with stdio (in this case, no data races are initiated, although no guarantees are given on the order in which extracted characters are attributed to threads).
Example
In below example explains about get_time function.
#include <iostream> #include <iomanip> #include <ctime> int main () { struct std::tm when; std::cout << "Please, enter the time: "; std::cin >> std::get_time(&when,"%R"); if (std::cin.fail()) std::cout << "Error reading time\n"; else { std::cout << "The time entered is: "; std::cout << when.tm_hour << " hours and " << when.tm_min << " minutes\n"; } return 0; }