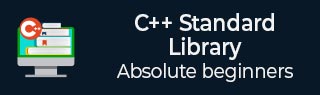
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Memory::get_deleter
C++ Memory::get_deleter is helpful for libraries that create shared ptr instances for client code. When the client passes a shared ptr back, the library needs to know whether the shared ptr came from the client or the library, and if it did, it needs to access the private data that was stored in the deleter when the instance was created.
Syntax
Following is the syntax for C++ Memory::get_deleter −
D* get_deleter (const shared_ptr<T>& sp) noexcept;
Parameters
sp − Its a shared pointer.
Example 1
Let's look into the following example, where we are going to use the get_deleter and getting the output.
#include <iostream> #include <memory> int main(){ std::shared_ptr<int> iptr(new int(999), [](int*p) { delete(p); }); auto _d = std::get_deleter<void(*)(int*)>(iptr); if(_d) std::cout<<"0"; else std::cout<<"1"; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
1
Example 2
Following is the another example, where we are going to use the get_deleter and retriveing the output.
#include <iostream> #include <memory> struct D { void operator()(int* p){ std::cout << "Welcome To Tutorialspoint\n"; delete[] p; } }; int main (){ std::shared_ptr<int> foo (new int[11],D()); int * bar = new int[20]; return 0; }
Output
On running the above code, it will display the output as shown below −
Welcome To Tutorialspoint
Example 3
let's look into the another scenario, where we are going to use the get_deleter and retriveing the output.
#include <iostream> #include <memory> struct A { A(){ std::cout << "HELLO\n"; } }; struct B { void operator()(A* p) const{ std::cout << "WORLD\n"; delete p; } }; int main(){ std::unique_ptr<A, B> up(new A(), B()); B& del = up.get_deleter(); }
Output
when the code gets executed, it will generate the output as shown below −
HELLO WORLD