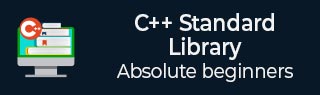
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Functional Library - equal_to
Description
It is a function object class for equality comparison and binary function object class whose call returns whether its two arguments compare equal (as returned by operator ==).
Declaration
Following is the declaration for std::equal_to.
template <class T> struct equal_to;
C++11
template <class T> struct equal_to;
Parameters
T − It is a type of the arguments and return type of the functional call.
Return Value
none
Exceptions
noexcep − It doesn't throw any exceptions.
Example
In below example explains about std::equal_to.
#include <iostream> #include <utility> #include <functional> #include <algorithm> int main () { std::pair<int*,int*> ptiter; int foo[]={10,20,30,40}; int bar[]={10,50,40,80}; ptiter = std::mismatch (foo, foo+5, bar, std::equal_to<int>()); std::cout << "First mismatching pair is: " << *ptiter.first; std::cout << " and " << *ptiter.second << '\n'; return 0; }
Let us compile and run the above program, this will produce the following result −
First mismatching pair is: 20 and 50
functional.htm
Advertisements