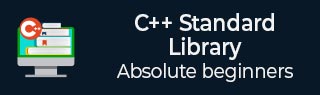
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Forward_list Library - operator>= Function
Description
The C++ function std::forward_list::operator>= tests whether first forward_list is greater than or equal to other or not.
Declaration
Following is the declaration for std::forward_list::operator>= function form std::forward_list header.
C++11
template <class T, class Alloc> bool operator>= (const forward_list<T,Alloc>& first, const forward_list<T,Alloc>& second);
Parameters
first − First forward_list object.
second − Second forward_list object of same type.
Return value
Returns true if first forward_list is greater than or equal to second otherwise false.
Exceptions
This member function never throws exception.
Time complexity
Linear i.e. O(n)
Example
The following example shows the usage of std::forward_list::operator>= function.
#include <iostream> #include <forward_list> using namespace std; int main(void) { forward_list<int> fl1 = {1, 2, 3, 4, 5}; forward_list<int> fl2 = {1, 2, 3, 4, 5}; if (fl1 >= fl2) cout << "First list is greater than or equal to second." << endl; fl2.push_front(6); if (!(fl1 >= fl2)) cout << "First list is not greater than or equal to second." << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
First list is greater than or equal to second. First list is not greater than or equal to second.
forward_list.htm
Advertisements