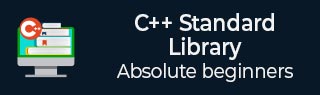
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Forward_list::cend() Function
The C++ std::forward_list::cend() function is used to retrieve a constant iterator to the element following the last element of the forward_list container.
The elements of this forward_list act as a placeholder; attempting to access it results in undefined behavior. In C++, an iterator is an object that can iterate over the elements in an STL(standard template library) container and provide access to an individual element. The main advantage of the iterator is to provide a common interface for all the containers type.
Syntax
Following is the syntax of the C++ std::forward_list::cend() function −
const_iterator cend() const;
Parameters
- It does not accept any parameter.
Return value
This function return a constant iterator to the element following the last element.
Example 1
If the forward_list is an int type, the cend() function returns a constant iterator to the element following the last element of the container.
In the following program, we are using the C++ std::forward_list::cend() function to retrieve a constant iterator to the element following the last element of the current forward_list {1, 2, 3, 4, 5}.
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<int> num_list = {1, 2, 3, 4, 5}; cout<<"The num_list contents are: "<<endl; for(int n : num_list){ cout<<n<<endl; } //using the cend() function auto it = num_list.cend(); cout<<"The constant iterator following the last element is: "<<*it; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The num_list contents are: 1 2 3 4 5 Segmentation fault
Example 2
Using the for loop along with the cend() function to retrieve a constant iterator of all the elements following the last element of the container.
Following is another example of the C++ std::forward_list::cend() function. Here, we are creating a forward_list(type char) named char_list with contents {'a', 'b', 'c', 'd' 'e'}. Then, we are using the for loop along with the cend() function to loop through the container and retrieve the constant iterator of all the elements following the last element of this forward_list.
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<char> char_list = {'a', 'b', 'c', 'd', 'e'}; int size = distance(char_list.begin(), char_list.end()); cout<<"The size of the char_list forward_list is: "<<size<<endl; //using cend() function cout<<"The constant iterator of all the elements are: "<<endl; for(auto it = char_list.begin(); it != char_list.cend(); it++){ cout<<*it<<endl; } }
Output
Following is the output of the above program −
The size of the char_list forward_list is: 5 The constant iterator of all the elements are: a b c d e
Example 3
Apart from the int type and char type forward_list element, we can also retrieve an iterator of the string type forward_list element.
In this program, we are creating a forward_list(type string) named languages with contents {"HTML", "CSS", "JavaScript", "C++", "Java"}. Then, we use the for loop along with the cend() function to loop through the container and retrieve the constant iterator of all the elements following the last element of this forward_list.
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<string> languages = {"HTML", "CSS", "JavaScript", "C++", "Java"}; int size = distance(languages.begin(), languages.end()); cout<<"The size of the vowels forward_list is: "<<size<<endl; //using cend() function cout<<"The constant iterators of an elements are: "<<endl; for(auto it = languages.begin(); it != languages.cend(); it++){ cout<<*it<<endl; } }
Output
This will generate the following output −
The size of the vowels forward_list is: 5 The constant iterators of an elements are: HTML CSS JavaScript C++ Java