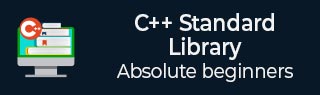
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Deque::push_front() Function
The C++ std::deque::push_front() function is used to insert the element at the beginning of the deque. It efficiently adds the element to the front, moving the existing elements to insert the new element. Unlike vector, deque supports efficient insertion and deletion at both the ends.
This function has 2 polymorphic variants: with using the default version and the move version (you can find the syntaxes of all the variants below).
Syntax
Following is the syntax for std::deque::push_front() function.
void push_front (const value_type& val); or void push_front (value_type&& val);
Parameters
- val − It indicates the value of the element to be inserted into the deque.
Return value
It does not return anything.
Exceptions
This function never throws exception.
Time complexity
The time complexity of this function is Constant i.e. O(1)
Example
In the following example, we are going to consider the basic usage of the push_front() function.
#include <iostream> #include <deque> int main() { std::deque<char> a = {'B', 'C', 'D'}; a.push_front('A'); for (auto& elem : a) { std::cout << elem << " "; } std::cout << std::endl; return 0; }
Output
Output of the above code is as follows −
A B C D
Example
Consider the following example, where we are going to insert the elements in the loop.
#include <iostream> #include <deque> int main() { std::deque<int> a; for (int x = 0; x <= 4; ++x) { a.push_front(x * 2); } for (auto y = a.begin(); y != a.end(); ++y) { std::cout << *y << " "; } std::cout << std::endl; return 0; }
Output
Following is the output of the above code −
8 6 4 2 0
Example
Let's look at the following example, where we are going to use push_front() with strings.
#include <iostream> #include <deque> #include <string> int main() { std::deque<std::string> a; a.push_front("Hi"); a.push_front("Hello"); for (const auto& str : a) { std::cout << str << " "; } std::cout << std::endl; return 0; }
Output
If we run the above code it will generate the following output −
Hello Hi