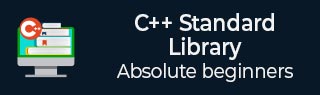
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ STL Library Cheat Sheet
- C++ STL - Cheat Sheet
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Deque::operator=() Function
The C++ std::deque::operator=() function is used to assign the contents of one deque to another. It replaces the contents of the target deque with the contents of the source deque. This function has 3 polymorphic variants: with using the copy version, move version and initializer list version (you can find the syntaxes of all the variants below).
The operator= can handle self-assignment correctly, meaning x = x will not cause issues.
Syntax
Following is the syntax for std::deque::operator=().
deque& operator= (const deque& x); or deque& operator= (deque&& x); or deque& operator= (initializer_list<value_type> il);
Parameters
- x − It indicates the deque object of the same type.
- il − It indicates an inititalizer_list object.
Return value
It returns this pointer
Exceptions
This function never throws exception.
Time complexity
The time complexity of this function is linear i.e. O(n)
Example
Let's look at the following example, where we are going to assign one deque to another.
#include <iostream> #include <deque> int main() { std::deque<char> x = {'A', 'B', 'C', 'D'}; std::deque<char> y; y = x; std::cout << "y contains: "; for (char elem : y) { std::cout << elem << " "; } return 0; }
Output
Output of the above code is as follows −
y contains: A B C D
Example
Consider the following example, where we are going to perform the self-assignment.
#include <iostream> #include <deque> int main() { std::deque<int> x = {11, 22, 3, 4}; x = x; std::cout << "x contains: "; for (int elem : x) { std::cout << elem << " "; } return 0; }
Output
Following is the output of the above code −
x contains: 11 22 3 4
Example
In the following example, we are going to assign the smaller deque to larger deque and observing the output.
#include <iostream> #include <deque> int main() { std::deque<char> x = {'A', 'B', 'C'}; std::deque<char> y = {'D', 'E', 'F', 'G', 'H'}; y = x; std::cout << "y contains: "; for (char elem : y) { std::cout << elem << " "; } return 0; }
Output
If we run the above code it will generate the following output −
y contains: A B C