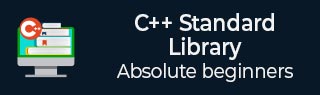
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ basic_ios Library - operator>>
Description
It is used to extract formatted input.
This operator (>>) applied to an input stream is known as extraction operator −
arithmetic types − Extracts and parses characters sequentially from the stream to interpret them as the representation of a value of the proper type, which is stored as the value of val. Internally, the function accesses the input sequence by first constructing a sentry object (with noskipws set to false). Then (if good), it calls num_get::get (using the stream's selected locale) to perform both the extraction and the parsing operations, adjusting the internal state flags accordingly. Finally, it destroys the sentry object before returning.
stream buffers − Extracts as many characters as possible from the stream and inserts them into the output sequence controlled by the stream buffer object pointed by sb (if any), until either the input sequence is exhausted or the function fails to insert into the object pointed by sb.
manipulators − Calls pf(*this), where pf may be a manipulator. Manipulators are functions specifically designed to be called when used with this operator. This operation has no effect on the input sequence and extracts no characters (unless the manipulator itself does, like ws).
Declaration
Following is the declaration for std::basic_istream::operator>>
C++98
arithmetic types (1) basic_istream& operator>> (bool& val); basic_istream& operator>> (short& val); basic_istream& operator>> (unsigned short& val); basic_istream& operator>> (int& val); basic_istream& operator>> (unsigned int& val); basic_istream& operator>> (long& val); basic_istream& operator>> (unsigned long& val); basic_istream& operator>> (float& val); basic_istream& operator>> (double& val); basic_istream& operator>> (long double& val); basic_istream& operator>> (void*& val); stream buffers (2) basic_istream& operator>> (basic_streambuf<char_type,traits_type>* sb ); manipulators (3) basic_istream& operator>> (basic_istream& (*pf)(basic_istream&)); basic_istream& operator>> ( basic_ios<char_type,traits_type>& (*pf)(basic_ios<char_type,traits_type>&)); basic_istream& operator>> (ios_base& (*pf)(ios_base&));
C++11
arithmetic types (1) basic_istream& operator>> (bool& val); basic_istream& operator>> (short& val); basic_istream& operator>> (unsigned short& val); basic_istream& operator>> (int& val); basic_istream& operator>> (unsigned int& val); basic_istream& operator>> (long& val); basic_istream& operator>> (unsigned long& val); basic_istream& operator>> (long long& val); basic_istream& operator>> (unsigned long long& val); basic_istream& operator>> (float& val); basic_istream& operator>> (double& val); basic_istream& operator>> (long double& val); basic_istream& operator>> (void*& val); stream buffers (2) basic_istream& operator>> (basic_streambuf<char_type,traits_type>* sb ); manipulators (3) basic_istream& operator>> (basic_istream& (*pf)(basic_istream&)); basic_istream& operator>> ( basic_ios<char_type,traits_type>& (*pf)(basic_ios<char_type,traits_type>&)); basic_istream& operator>> (ios_base& (*pf)(ios_base&));
Parameters
- val − Object where the value that the extracted characters represent is stored. Notice that the type of this argument (along with the stream's format flags) influences what constitutes a valid representation.
sb − Pointer to a basic_streambuf object on whose controlled output sequence the characters are copied.
pf − A function that takes and returns a stream object.
Return Value
Returns the basic_istream object (*this).
Exceptions
Basic guarantee − if an exception is thrown, the object is in a valid state.
Data races
Modifies val or the object pointed by sb.
Example
In below example for std::basic_istream::operator>>
#include <iostream> int main () { int n; std::cout << "Enter a number: "; std::cin >> n; std::cout << "You have entered: " << n << '\n'; std::cout << "Enter a hexadecimal number: "; std::cin >> std::hex >> n; std::cout << "Its decimal equivalent is: " << n << '\n'; return 0; }
Let us compile and run the above program, this will produce the following result −
Enter a number: 11 You have entered: 11 Enter a hexadecimal number: 5E Its decimal equivalent is: 94