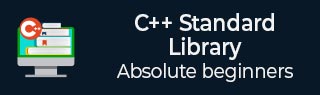
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ basic_istream Library - operator>>
Description
This operator (>>) applied to an input stream is known as extraction operator.
Declaration
Following is the declaration for std::basic_istream::operator>>.
C++98
basic_istream& operator>> (bool& val); basic_istream& operator>> (short& val); basic_istream& operator>> (unsigned short& val); basic_istream& operator>> (int& val); basic_istream& operator>> (unsigned int& val); basic_istream& operator>> (long& val); basic_istream& operator>> (unsigned long& val); basic_istream& operator>> (float& val); basic_istream& operator>> (double& val); basic_istream& operator>> (long double& val); basic_istream& operator>> (void*& val); stream buffers (2) basic_istream& operator>> (basic_streambuf<char_type,traits_type>* sb ); manipulators (3) basic_istream& operator>> (basic_istream& (*pf)(basic_istream&)); basic_istream& operator>> ( basic_ios<char_type,traits_type>& (*pf)(basic_ios<char_type,traits_type>&)); basic_istream& operator>> (ios_base& (*pf)(ios_base&));
C++11
basic_istream& operator>> (bool& val); basic_istream& operator>> (short& val); basic_istream& operator>> (unsigned short& val); basic_istream& operator>> (int& val); basic_istream& operator>> (unsigned int& val); basic_istream& operator>> (long& val); basic_istream& operator>> (unsigned long& val); basic_istream& operator>> (long long& val); basic_istream& operator>> (unsigned long long& val); basic_istream& operator>> (float& val); basic_istream& operator>> (double& val); basic_istream& operator>> (long double& val); basic_istream& operator>> (void*& val); stream buffers (2) basic_istream& operator>> (basic_streambuf<char_type,traits_type>* sb ); manipulators (3) basic_istream& operator>> (basic_istream& (*pf)(basic_istream&)); basic_istream& operator>> ( basic_ios<char_type,traits_type>& (*pf)(basic_ios<char_type,traits_type>&)); basic_istream& operator>> (ios_base& (*pf)(ios_base&));
Parameters
val − It is an object where the value that the extracted characters represent is stored.
sb − Pointer to a basic_streambuf object on whose controlled output sequence the characters are copied.
pf − A function that takes and returns a stream object. It generally is a manipulator function.
Return Value
Returns the basic_istream object (*this).
Exceptions
Modifies val or the object pointed by sb and modifies the stream object.
Exception safety
Basic guarantee − if an exception is thrown, the object is in a valid state.
Example
In below example for std::basic_istream::operator>>
#include <iostream> int main () { int n; std::cout << "Enter a number: "; std::cin >> n; std::cout << "You have entered: " << n << '\n'; std::cout << "Enter a hexadecimal number: "; std::cin >> std::hex >> n; std::cout << "Its decimal equivalent is: " << n << '\n'; return 0; }
Let us compile and run the above program, this will produce the following result −
Enter a number: 1 You have entered: 1 Enter a hexadecimal number: 5E Its decimal equivalent is: 94