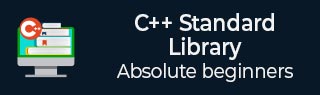
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Atomic Library - Fetch Add
Description
It adds a non-atomic value to an atomic object and obtains the previous value of the atomic.
Declaration
Following is the declaration for std::atomic_fetch_add.
template< class Integral > Integral atomic_fetch_add( std::atomic<Integral>* obj, Integral arg );
C++11
template< class Integral > Integral atomic_fetch_add( volatile std::atomic<Integral>* obj, Integral arg );
Following is the declaration for std::atomic_fetch_add_explicit.
template< class T > T* atomic_fetch_add_explicit( volatile std::atomic<T*>* obj, std::ptrdiff_t arg, std::memory_order order );
C++11
template< class T > T* atomic_fetch_add_explicit( std::atomic<T*>* obj, std::ptrdiff_t arg, std::memory_order order );
Parameters
obj − It is used in pointer to the atomic object to modify.
desr − It is used to store the value in the atomic object.
order − It is used to synchronise the memory ordering for this operation.
Return Value
It returns the value immediately preceding the effects of this function in the modification order of *obj.
Exceptions
No-noexcept − this member function never throws exceptions.
Example
In below example for std::atomic_fetch_add_explicit.
#include <string> #include <thread> #include <vector> #include <iostream> #include <atomic> #include <chrono> const int N = 10; std::atomic<int> cnt = ATOMIC_VAR_INIT(N); std::vector<int> data; void reader(int id) { for(;;) { while(std::atomic_fetch_sub(&cnt, 1) <= 0) std::atomic_fetch_add(&cnt, 1); if(!data.empty()) std::cout << ( "reader " + std::to_string(id) + " sees " + std::to_string(*data.rbegin()) + '\n'); if(data.size() == 100) break; std::atomic_fetch_add(&cnt, 1); std::this_thread::sleep_for(std::chrono::milliseconds(1)); } } void writer() { for(int n = 0; n < 100; ++n) { while(std::atomic_fetch_sub(&cnt, N+1) != N) std::atomic_fetch_add(&cnt, N+1); data.push_back(n); std::cout << "writer pushed back " << n << '\n'; std::atomic_fetch_add(&cnt, N+1); std::this_thread::sleep_for(std::chrono::milliseconds(1)); } } int main() { std::vector<std::thread> v; for (int n = 0; n < N; ++n) { v.emplace_back(reader, n); } v.emplace_back(writer); for (auto& t : v) { t.join(); } }
The output should be like this −
writer pushed back 0 reader 8 sees 0 reader 3 sees 0 reader 1 sees 0 ............... reader 2 sees 99 reader 6 sees 99 reader 1 sees 99
atomic.htm
Advertisements