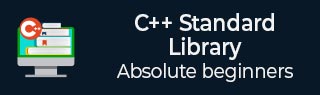
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Array Library - operator<=() Function
Description
The C++ function bool operator>() compares two array container elements sequentially. Comparision stops at first mismatch or when container elements are exhuasted. For comparision size and data type of both container must be same otherwise compiler will report compilation error.
Declaration
Following is the declaration for bool operator>() function form std::array header.
template <class T, size_t N> bool operator> ( const array<T,N>& arr1, const array<T,N>& arr2 );
Parameters
arr1 and arr2 − two array containers of same size and type.
Return Value
Returns true if first array container is greater than second otherwise false.
Exceptions
This function never throws exception.
Time complexity
Linear i.e. O(n)
Example
The following example shows the usage of bool operator>() function.
#include <iostream> #include <array> using namespace std; int main(void) { array<int, 5> arr1 = {1, 2, 3, 4, 5}; array<int, 5> arr2 = {1, 2, 4, 3, 5}; bool result; result = (arr2 > arr1); if (result == true) cout << "arr2 is greater than arr1\n"; else cout << "arr1 is greater that arr2\n"; return 0; }
Let us compile and run the above program, this will produce the following result −
arr2 is greater than arr1
array.htm
Advertisements