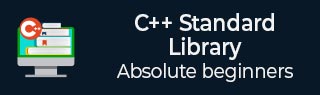
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Array Library - end() Function
Description
The C++ function std::array::end() returns an iterator which points to the past-end element of array.
Declaration
Following is the declaration for std::array::end() function form std::array header.
iterator end() noexcept; const_iterator end() noexcept;
Parameters
None
Return Value
Returns an iterator pointing to the past-the-end element in the array. This element act as a place-holder and never stores the actual data that is why deferencing this location would result undefined behavior.
If array object is const-qualified, method return const iterator otherwise returns iterator.
Exceptions
This member function never throws exception.
Time complexity
Constant i.e. O(1)
Example
The following example shows the usage of std::array::end() function.
#include <iostream> #include <array> using namespace std; int main(void) { array<int, 5> arr = {10, 20, 30, 40, 50}; /* iterator pointing at the start of array */ auto start = arr.begin(); /* iterator pointing past−the−end of array */ auto end = arr.end(); /* iterate complete array */ while (start < end) { cout << *start << " "; ++start; } cout << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
10 20 30 40 50
array.htm
Advertisements