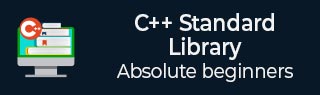
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Algorithm Library - generate_n() Function
Description
The C++ function std::algorithm::generate_n() assigns the value returned by successive calls to gen to the first n elements of the sequence pointed by the first.
Declaration
Following is the declaration for std::algorithm::generate_n() function form std::algorithm header.
C++98
template <class OutputIterator, class Size, class Generator> void generate_n(OutputIterator first, Size n, Generator gen);
Parameters
first − Output iterator to the initial position.
n − Number of values to generate.
gen − Generator function that is called with no arguments and returns some value.
Return value
None
Exceptions
Throws exception if either gen function or the element assignment or an operation on an iterator throws exception.
Please note that invalid parameters cause undefined behavior.
Time complexity
Linear.
Example
The following example shows the usage of std::algorithm::generate_n() function.
#include <iostream> #include <algorithm> using namespace std; int main(void) { int arr[10] = {0}; /* assign value to only first 5 elements */ generate_n(arr, 5, rand); cout << "First five random numbers are" << endl; for (int i = 0; i < 10; ++i) cout << arr[i] << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
First five random numbers are 1804289383 846930886 1681692777 1714636915 1957747793 0 0 0 0 0