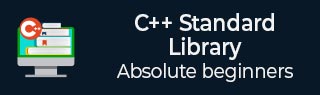
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <multimap>
- C++ Library - <queue>
- C++ Library - <priority_queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Numeric Library - adjacent_difference
Description
It computes adjacent difference of range.
Declaration
Following is the declaration for std::adjacent_difference.
C++98
template <class InputIterator, class OutputIterator> OutputIterator adjacent_difference (InputIterator first, InputIterator last, OutputIterator result); template <class InputIterator, class OutputIterator, class BinaryOperation> OutputIterator adjacent_difference ( InputIterator first, InputIterator last, OutputIterator result, BinaryOperation binary_op );
C++11
template <class InputIterator, class OutputIterator> OutputIterator adjacent_difference (InputIterator first, InputIterator last, OutputIterator result); template <class InputIterator, class OutputIterator, class BinaryOperation> OutputIterator adjacent_difference ( InputIterator first, InputIterator last, OutputIterator result, BinaryOperation binary_op );
first, last − It iterators to the initial and final positions in a sequence.
init − It is an initial value for the accumulator.
binary_op − It is binary operation.
Return Value
It returns an iterator pointing to past the last element of the destination sequence where resulting elements have been stored.
Exceptions
It throws if any of binary_op, the assignments or an operation on an iterator throws.
Data races
The elements in the range [first1,last1) are accessed.
Example
In below example for std::adjacent_difference.
#include <iostream> #include <functional> #include <numeric> int myop (int x, int y) {return x+y;} int main () { int val[] = {10,20,30,50,60,70}; int result[7]; std::adjacent_difference (val, val+7, result); std::cout << "Default adjacent_difference: "; for (int i=0; i<7; i++) std::cout << result[i] << ' '; std::cout << '\n'; std::adjacent_difference (val, val+7, result, std::multiplies<int>()); std::cout << "Functional operation multiplies: "; for (int i=0; i<7; i++) std::cout << result[i] << ' '; std::cout << '\n'; std::adjacent_difference (val, val+7, result, myop); std::cout << "Custom function operation: "; for (int i=0; i<7; i++) std::cout << result[i] << ' '; std::cout << '\n'; return 0; }
The output should be like this −
Default adjacent_difference: 10 10 10 20 10 10 4197079 Functional operation multiplies: 10 200 600 1500 3000 4200 293800430 Custom function operation: 10 30 50 80 110 130 4197219
numeric.htm
Advertisements