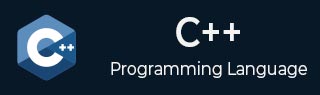
- C++ Basics
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Data Types
- C++ Variable Types
- C++ Variable Scope
- C++ Constants/Literals
- C++ Modifier Types
- C++ Storage Classes
- C++ Operators
- C++ Loop Types
- C++ Decision Making
- C++ Functions
- C++ Numbers
- C++ Arrays
- C++ Strings
- C++ Pointers
- C++ References
- C++ Date & Time
- C++ Basic Input/Output
- C++ Data Structures
- C++ Object Oriented
- C++ Classes & Objects
- C++ Inheritance
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Advanced
- C++ Files and Streams
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
C++ Interview Questions
Dear readers, these C++ Interview Questions have been designed specially to get you acquainted with the nature of questions you may encounter during your interview for the subject of C++. As per my experience good interviewers hardly plan to ask any particular question during your interview, normally questions start with some basic concept of the subject and later they continue based on further discussion and what you answer −
Object Oriented Programming System.
Class is a blue print which reflects the entities attributes and actions. Technically defining a class is designing an user defined data type.
An instance of the class is called as object.
Single, Multilevel, Multiple, Hierarchical and Hybrid.
If a class member is protected then it is accessible in the inherited class. However, outside the both the private and protected members are not accessible.
The process of binding the data and the functions acting on the data together in an entity (class) called as encapsulation.
Abstraction refers to hiding the internal implementation and exhibiting only the necessary details.
Inheritance is the process of acquiring the properties of the exiting class into the new class. The existing class is called as base/parent class and the inherited class is called as derived/child class.
Declaring a variable volatile directs the compiler that the variable can be changed externally. Hence avoiding compiler optimization on the variable reference.
A function prefixed with the keyword inline before the function definition is called as inline function. The inline functions are faster in execution when compared to normal functions as the compiler treats inline functions as macros.
Storage class specifies the life or scope of symbols such as variable or functions.
The following are storage classes supported in C++
auto, static, extern, register and mutable
A constant class object’s member variable can be altered by declaring it using mutable storage class specifier. Applicable only for non-static and non-constant member variable of the class.
Shallow copy does memory dumping bit-by-bit from one object to another. Deep copy is copy field by field from object to another. Deep copy is achieved using copy constructor and or overloading assignment operator.
A virtual function with no function body and assigned with a value zero is called as pure virtual function.
A class with at least one pure virtual function is called as abstract class. We cannot instantiate an abstract class.
A reference variable is an alias name for the existing variable. Which mean both the variable name and reference variable point to the same memory location. Therefore updation on the original variable can be achieved using reference variable too.
A static variable does exit though the objects for the respective class are not created. Static member variable share a common memory across all the objects created for the respective class. A static member variable can be referred using the class name itself.
A static member function can be invoked using the class name as it exits before class objects comes into existence. It can access only static members of the class.
wchar_t
Dot (.) and Arrow ( -> )
No, Defining a class/structure is just a type definition and will not allocated memory for the same.
bool, is the new primitive data type introduced in C++ language.
Defining several functions with the same name with unique list of parameters is called as function overloading.
Defining a new job for the existing operator w.r.t the class objects is called as operator overloading.
No, it’s a class from STL (Standard template library).
cin, cout, cerr and clog.
Private & Protected.
Scope resolution operator (::)
A destructor is the member function of the class which is having the same name as the class name and prefixed with tilde (~) symbol. It gets executed automatically w.r.t the object as soon as the object loses its scope. It cannot be overloaded and the only form is without the parameters.
A constructor is the member function of the class which is having the same as the class name and gets executed automatically as soon as the object for the respective class is created.
Every class does have a constructor provided by the compiler if the programmer doesn’t provides one and known as default constructor. A programmer provided constructor with no parameters is called as default constructor. In such case compiler doesn’t provides the constructor.
‘new’ is the operator can be used for the same.
‘delete’ operator is used to release the dynamic memory which was created using ‘new’ operator.
Yes, as C is the subset of C++, we can all the functions of C in C++ too.
No, we need to use free() of C language for the same.
A function which is not a member of the class but still can access all the member of the class is called so. To make it happen we need to declare within the required class following the keyword ‘friend’.
A copy constructor is the constructor which take same class object reference as the parameter. It gets automatically invoked as soon as the object is initialized with another object of the same class at the time of its creation.
C++ does supports exception handling. try, catch & throw are keyword used for the same.
This, is the pointer variable of the compiler which always holds the current active object’s address.
By default the members of struct are public and by default the members of the class are private.
Yes.
A variable whose scope is applicable only within a block is said so. Also a variable in C++ can be declared anywhere within the block.
If the file already exists, its content will be truncated before opening the file.
The scope resolution operator is used to
- Resolve the scope of global variables.
- To associate function definition to a class if the function is defined outside the class.
A namespace is the logical division of the code which can be used to resolve the name conflict of the identifiers by placing them under different name space.
The arguments/parameters which are sent to the main() function while executing from the command line/console are called so. All the arguments sent are the strings only.
A template class is a generic class. The keyword template can be used to define a class template.
The catch block with ellipses as follows
catch(…) { }
By default every local variable of the function is automatic (auto). In the below function both the variables ‘i’ and ‘j’ are automatic variables.
void f() { int i; auto int j; }
NOTE − A global variable can’t be an automatic variable.
A static local variables retains its value between the function call and the default value is 0. The following function will print 1 2 3 if called thrice.
void f() { static int i; ++i; printf(“%d “,i); }
If a global variable is static then its visibility is limited to the same source code.
Used to resolve the scope of global symbol
#include <iostream> using namespace std; main() { extern int i; cout<<i<<endl; } int i = 20;
The starting address of the array is called as the base address of the array.
If a variable is used most frequently then it should be declared using register storage specifier, then possibly the compiler gives CPU register for its storage to speed up the look up of the variable.
Yes, it can be but cannot be executed, as the execution requires main() function definition.
Every local variable by default being an auto variable is stored in stack memory
A class containing at least one member variable of another class type in it is called so.
A C++ program consists of various tokens and a token is either a keyword, an identifier, a constant, a string literal, or a symbol.
Preprocessor is a directive to the compiler to perform certain things before the actual compilation process begins.
The arguments which we pass to the main() function while executing the program are called as command line arguments. The parameters are always strings held in the second argument (below in args) of the function which is array of character pointers. First argument represents the count of arguments (below in count) and updated automatically by operating system.
main( int count, char *args[]) { }
Call by value − We send only values to the function as parameters. We choose this if we do not want the actual parameters to be modified with formal parameters but just used.
Call by address − We send address of the actual parameters instead of values. We choose this if we do want the actual parameters to be modified with formal parameters.
Call by reference − The actual parameters are received with the C++ new reference variables as formal parameters. We choose this if we do want the actual parameters to be modified with formal parameters.
Error, It is invalid that either of the operands for the modulus operator (%) is a real number.
Opiton –lm to be used as > g++ –lm <file.cpp>
No, there is no such provision available.
Bjarne Stroustrup.
sizeof
We can apply scope resolution operator (::) to the for the scope of global variable.
The only two permitted operations on pointers are
Comparision ii) Addition/Substraction (excluding void pointers)
Function calling itself is called as recursion.
Program name.
Ideally it is 32 characters and also implementation dependent.
By default the functions are called by value.
Public, private & protected
Delete[] is used to release the array allocated memory which was allocated using new[] and delete is used to release one chunk of memory which was allocated using new.
Not necessarily, a class having at least one pure virtual function is abstract class too.
No, it will be error as the compiler fails to do conversion.
The program shall quit abruptly.
No, exceptions can be handled whereas program cannot resolve errors.
Defining the functions within the base and derived class with the same signature and name where the base class’s function is virtual.
seekg()
seekp()
No, only the class member variables determines the size of the respective class object.
We can create an empty class and the object size will be 1.
Default namespace defined by C++.
Standard template library
cout is the object of ostream class. The stream ‘cout’ is by default connected to console output device.
cin is the object of istream class. The stream ‘cin’ is by default connected to console input device.
It is used to specify the namespace being used in.
Arrow (->) operator can be used for the same
If a header file is included with in < > then the compiler searches for the particular header file only with in the built in include path. If a header file is included with in “ “, then the compiler searches for the particular header file first in the current working directory, if not found then in the built in include path
S++, as it is single machine instruction (INC) internally.
The parameters sent to the function at calling end are called as actual parameters while at the receiving of the function definition called as formal parameters.
Declaration associates type to the variable whereas definition gives the value to the variable.
goto.
No, it contains invalid octal digits.
It will be used to undefine an existing macro definition.
No, we cannot.
A virtual destructor ensures that the objects resources are released in the reverse order of the object being constructed w.r.t inherited object.
The objects are destroyed in the reverse order of their creation.
A class members can gain accessibility over other class member by placing the class declaration prefixed with the keyword ‘friend’ in the destination class.
What is Next ?
Further you can go through your past assignments you have done with the subject and make sure you are able to speak confidently on them. If you are fresher then interviewer does not expect you will answer very complex questions, rather you have to make your basics concepts very strong.
Second it really doesn't matter much if you could not answer few questions but it matters that whatever you answered, you must have answered with confidence. So just feel confident during your interview. We at tutorialspoint wish you best luck to have a good interviewer and all the very best for your future endeavor. Cheers :-)