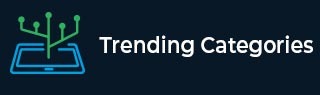
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ set for user define data type
Here we will see how we can make a set for user defined datatypes. The Set is present in C++ STL. This is a special type of data structure, it can store data in sorted order, and does not support duplicate entry. We can use set for any type of data, but here we will see how we can also use set for userdefined datatypes.
To use user-defined datatypes into stack, we have to override < operator, that can compare two values of that type. If this is not present, it cannot compare two objects, so the set cannot store data in sorted order, so will raise an exception.
Example
#include <iostream> #include<set> using namespace std; class Student { int id, marks; public: Student(int id, int marks){ this->id = id; this->marks = marks; } bool operator <(const Student& st) const{ //sort using id, return (this->id < st.id); } void display() const{ cout << "(" << id << ", " << marks << ")\n"; } }; main() { Student s1(5, 70), s2(3, 86), s3(2, 91), s4(2, 60), s5(1, 78), s6(6, 53), s7(4, 59); //the set will not consider duplicate id set<Student> st_set; st_set.insert(s1); st_set.insert(s2); st_set.insert(s3); st_set.insert(s4); st_set.insert(s5); st_set.insert(s6); st_set.insert(s7); set<Student>::iterator it; for(it = st_set.begin(); it != st_set.end(); it++){ it->display(); } }
Output
(1, 78) (2, 91) (3, 86) (4, 59) (5, 70) (6, 53)
Advertisements