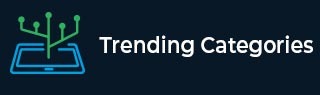
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ program to store student roll and name using map STL
Suppose we have a map data structure for students roll and name the roll is an integer data and name is string type data. In our standard input we provide n queries. In each query (in each line) there must be two elements and for type 1 query there are three elements. First one is the operator, second one is the roll and third one is the name, for two elements query the second item is the roll number. The operations are like below−
Insert. This will insert the name into the map at corresponding roll
Delete. This will delete the against the roll number from the map (if exists).
Search. This will search the name into the map with roll number, if present shows the name, otherwise show Not Found.
So, if the input is like n = 8, queries = [[1,5,"Atanu"], [1,8, "Tapan"], [1,3,"Manish"],[2,8],[1,9, "Piyali"], [3,8],[3,3], [3,5]], then the output will be [Not found, Manish, Atanu] because the roll 8 is not present, the name of student with roll 3 is Manish and name of student with roll 5 is "Atanu".
To solve this, we will follow these steps −
- n := the number of queries
- Define one map m, of integer type key and string type value.
- while n is non-zero, decrease n in each iteration, do:
- take current query type t
- take roll number
- if t is same as 1, then:
- take the name
- m[roll] := name
- otherwise when t is same as 2, then:
- m[roll] := a blank string
- otherwise
- if m[roll] is not blank string, then:
- display m[roll]
- Otherwise
- display "Not found"
- if m[roll] is not blank string, then:
Example
Let us see the following implementation to get better understanding −
#include <iostream> #include <map> using namespace std; int main(){ int n; cin >> n; map<int, string> m; while (n--) { int t; cin >> t; int roll; cin >> roll; if (t == 1) { string name; cin >> name; m[roll] = name; } else if (t == 2) { m[roll] = ""; } else { if(m[roll] != "") cout << m[roll] << endl; else cout << "Not found" << endl; } } }
Input
8 1 5 Atanu 1 8 Tapan 1 3 Manish 2 8 1 9 Piyali 3 8 3 3 3 5
Output
Not found Manish Atanu