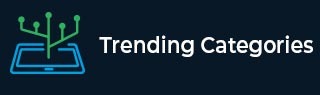
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ program to rearrange all elements of array which are multiples of x in increasing order
We are given an integer type array as ‘int arr[]’ and an integer type variable as ‘x’. The task is to rearrange all the elements of an array in such a manner that they will be divisible by a given integer value ‘x’ and the arrangement should be in an increasing order.
Let us see various input output scenarios for this −
Input − int arr[] = {4,24, 3, 5, 7, 22, 12, 10}, int x = 2
Output − Rearrangement of all elements of array which are multiples of x 2 in decreasing order is: 4 10 3 5 7 12 22 24
Explanation −we are given an integer type array containing values as {4,24, 3, 5, 7, 22, 12, 10} and x with the value as 2. Now firstly we will check all the elements from an array which are divisible by 2 i.e. 4, 24, 22, 12, 10. Now we will arrange all the elements in an increasing orderi.e. 4, 10, 3, 5, 7, 12, 22, 24 which is the final output.
Input − nt arr[] = {4,24, 3, 5, 7, 22, 12, 10}, int x = 3
Output − Rearrangement of all elements of array which are multiples of x 3 in decreasing order is: 4 3 12 5 7 22 24 10
Explanation − we are given an integer type array containing values as {4,24, 3, 5, 7, 22, 12, 10} and x with the value as 3. Now firstly we will check all the elements from an array which are divisible by 3 i.e. 3, 24, 12. Now we will arrange all the elements in an increasing order i.e. 4, 3, 12, 5, 7, 22, 24, 10 which is the final output.
Approach used in the below program is as follows
Declare an integer type array. Calculate the size of an array and store it in a variable named size. Declare an integer type variable ‘x’ against which we need to rearrange an array.
Pass the data to the function Rearrange_Elements(arr, size, x)
Inside the function Rearrange_Elements(arr, size, x)
Create a variable as vec of type vector storing integer type values.
Start loop FOR from i to 0 till i less than size. Inside the loop, check IF arr[i] % x = 0 then push arr[i] to the vec
Sort an array using C++ STL sort method where we will pass begin() and end() as a parameter to the function.
Start loop FOR from i to 0 till i less than size. Check IF arr[i] % x = 0 then set arr[i] to vec[j++].
Print an array using the for loop by traversing it from the first element of an array till the last available element.
Example
#include <bits/stdc++.h> using namespace std; void Rearrange_Elements(int arr[], int size, int x){ vector<int> vec; int j = 0; for(int i = 0; i < size; i++){ if(arr[i] % x == 0){ vec.push_back(arr[i]); } } sort(vec.begin(), vec.end()); for (int i = 0; i < size; i++){ if(arr[i] % x == 0){ arr[i] = vec[j++]; } } cout<<"Rearrangement of all elements of array which are multiples of x "<<x<<" in decreasing order is: "; for(int i = 0; i < size; i++){ cout << arr[i] << " "; } } int main(){ int arr[] = {4,24, 3, 5, 7, 22, 12, 10}; int x = 2; int size = sizeof(arr) / sizeof(arr[0]); Rearrange_Elements(arr, size, x); return 0; }
Output
If we run the above code it will generate the following Output
Rearrangement of all elements of array which are multiples of x 2 in decreasing order is: 4 10 3 5 7 12 22 24