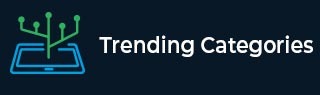
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ program to print values of different data types provided as input
Suppose we are given an integer value, a long value, a character value, a float value, and a double value as inputs. We have to print the values that are given to us as input maintaining their precision.
So, if the input is like integer value = 15, long value = 59523256297252, character value = 'y', float value = 367.124, double value = 6464292.312621, then the output will be
15 59523256297252 y 367.124 6464292.31262
To solve this, we will follow these steps −
- Print the values given as input in separate lines.
Example
Let us see the following implementation to get better understanding −
#include <iostream> #include <cstdio> using namespace std; void solve(int a, long b, char c, float d, double e) { cout << a << endl << b << endl << c << endl << d << endl; printf("%.5f", e); } int main() { solve(15, 59523256297252, 'y', 367.124, 6464292.312621); return 0; }
Input
15, 59523256297252, 'y', 367.124, 6464292.312621
Output
15 59523256297252 y 367.124 6464292.31262
Advertisements