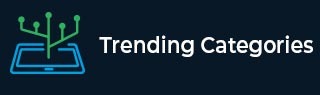
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ program to print values in a specified format
Suppose we are given three double values. We have to format them and print them in the following format.
We have to print the integer part of the first value in hexadecimal format with lowercase letters.
We have to print the second value up to two decimal places, putting a sign before it to show whether it is positive or negative. The second value to be printed has to be rightjustified and 15 characters long, padded with underscores in the left unused positions.
We have to print the third value up to nine decimal places in a scientific notation.
So, if the input is like 256.367, 5783.489, 12.5643295643, then the output will be
0x100 _______+5783.49 1.256432956E+01
To solve this, we will follow these steps −
The hex flag prints the value in hexadecimal format, the showbase flag shows the '0x'prefix for hex values, the left flag pads the value to the output field with inserting fill characters to the right of the value, and the nouppercase flag prints the output in all lowercase letters.
The right flag pads the value to the output field with inserting fill characters to the left of the value, the fixed flag prints the values in a fixed-point notation, the set(15)sets the output field length to 15, the showpos flag inserts a '+' sign before the output, setfill('_') pads the output with underscores, and setprecision() sets the precison of the value up to 2 decimal places.
setprecision() sets the precison of the value up to 9 decimal places, the scientific flag prints the value in a scientific notation, uppercase makes the output value in all uppercase letters, and noshowpos omits any positive sign before the output value.
Let us see the following implementation to get better understanding −
#include <iostream> #include <iomanip> using namespace std; void solve(double a, double b, double c) { cout << hex << showbase << nouppercase << left << (long long) a << endl; cout << right << fixed << setw(15) << setfill('_') << setprecision(2) << showpos << b << endl; cout << setprecision(9) << scientific << uppercase << noshowpos << c << endl; } int main() { solve(256.367, 5783.489, 12.5643295643); return 0; }
Input
256.367, 5783.489, 12.5643295643
Output
0x100 _______+5783.49 1.256432956E+01