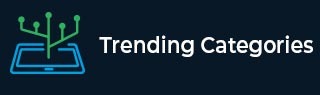
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program to Implement Levenshtein Distance Computing Algorithm
The Levenshtein distance between two strings means the minimum number of edits needed to transform one string into the other, with the edit operations i.e; insertion, deletion, or substitution of a single character.
For example: The Levenshtein Distance between cat and mat is 1 −
cat mat(substitution of ‘c’ with ‘m’)
Here is a C++ Program to implement Levenshtein Distance computing algorithm.
Algorithms
Begin Take the strings as input and also find their length. For i = 0 to l1 dist[0][i] = i For j = 0 to l2 dist[j][0] = j For j=1 to l1 For i=1 to l2 if(s1[i-1] == s2[j-1]) track= 0 else track = 1 done t = MIN((dist[i-1][j]+1),(dist[i][j-1]+1)) dist[i][j] = MIN(t,(dist[i-1][j-1]+track)) Done Done Print the Levinstein distance: dist[l2][l1] End
Example
#include <iostream> #include <math.h> #include <string.h> using namespace std; #define MIN(x,y) ((x) < (y) ? (x) : (y)) //calculate minimum between two values int main() { int i,j,l1,l2,t,track; int dist[50][50]; //take the strings as input char s1[] = "tutorials"; char s2[] = "point"; //stores the lenght of strings s1 and s2 l1 = strlen(s1); l2= strlen(s2); for(i=0;i<=l1;i++) { dist[0][i] = i; } for(j=0;j<=l2;j++) { dist[j][0] = j; } for (j=1;j<=l1;j++) { for(i=1;i<=l2;i++) { if(s1[i-1] == s2[j-1]) { track= 0; } else { track = 1; } t = MIN((dist[i-1][j]+1),(dist[i][j-1]+1)); dist[i][j] = MIN(t,(dist[i-1][j-1]+track)); } } cout<<"The Levinstein distance is:"<<dist[l2][l1]; return 0; }
Output
The Levinstein distance is:8
Advertisements