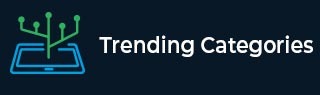
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program to get the subarray from an array using a specified range of indices
A sequence of memory sections is used to store homogeneous data in an array, a linear sequential data structure. Similar to other data structures, an array needs to have specific qualities to insert, delete, traverse, and update elements efficiently. In C++, our arrays are static. There are also a few dynamic array structures available in C++. In this article, we will see how to get a subarray from a bigger array using starting and ending indices in C++.
Understanding the concept with examples
Given array A = [10, 14, 65, 85, 96, 12, 35, 74, 69] Given two indices 2 and 7, it will pick elements [65, 85, 96, 12, 35] The picked elements are coming from index 2 to index 6, (excluding 7) of the given array A.
From the example, we can see an array and two indices are given to pick subarrays from those locations. We will see the solutions in different modes. First, find the basic solution using the traditional static array method. Let us see the algorithm along with the C++ implementation code below.
Algorithm
Take an array A as input and also take two indices i and j as input
create a blank array called Sub
for index k in range i to j, do
insert A [ k ] into sub
end for
return sub
Example
#include <iostream> # define Z 50 using namespace std; void displayArr(int arr[], int n){ for( int i = 0; i < n; i++ ){ cout << arr[ i ] << ", "; } cout << endl; } void pickSubarray( int A[], int n, int sub[], int &m, int i, int j ) { int ind = 0; for( int k = i; k < j; k++, ind++ ) { sub[ ind ] = A[ k ]; m += 1; } } int main() { int A[ Z ] = {57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14}; int n = 12; int sub[ Z ]; int m = 0; cout << "Given Array: "; displayArr( A, n ); cout << "Sub array from index 3 to 9: "; pickSubarray( A, n, sub, m, 3, 9); displayArr( sub, m ); }
Output
Given Array: 57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14, Sub array from index 3 to 9: 19, 86, 52, 32, 14, 76,
Here when we are copying the elements into the new array, the number of elements count m is also getting increased one by one to keep track of how many elements are there in the second array or the subarray.
Using Dynamic Arrays or Vectors
The same thing can be done using vectors. Vectors are dynamic arrays present inside C++ STL. If we consider vectors, we do not need to update the total size explicitly. Because vectors are dynamic, it updates automatically while inserting elements into them. The algorithm is the same, we are jumping to the implementation section.
Example
#include <iostream> #include <vector> # define Z 50 using namespace std; void displayArr( vector<int> v ){ for( int i = 0; i < v.size() ; i++ ){ cout << v[ i ] << ", "; } cout << endl; } vector<int> pickSubarray( vector<int> A, int i, int j ) { int ind = 0; vector<int> sub; for( int k = i; k < j; k++ ) { sub.push_back( A[ k ] ); } return sub; } int main() { vector<int> A = {57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14}; vector<int> sub; cout << "Given Array: "; displayArr( A ); cout << "Sub array from index 3 to 9: "; sub = pickSubarray( A, 3, 9); displayArr( sub ); }
Output
Given Array: 57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14, Sub array from index 3 to 9: 19, 86, 52, 32, 14, 76,
Using the vector iterators
The previous method is a manual process. However, we can do the same thing using the vector iterators where we just use the start and/or end pointers of vector elements and add the offset values which are the indices. It is another simpler solution directly using optimized C++ STL. Let us see the code for a clear understanding.
Example
#include <iostream> #include <vector> # define Z 50 using namespace std; void displayArr( vector<int> v ){ for( int i = 0; i < v.size() ; i++ ){ cout << v[ i ] << ", "; } cout << endl; } vector<int> pickSubarray( vector<int> A, int i, int j ) { auto first = A.begin() + i; auto last = A.begin() + j; vector<int> sub( first, last ); return sub; } int main() { vector<int> A = {57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14}; vector<int> sub; cout << "Given Array: "; displayArr( A ); cout << "Sub array from index 3 to 9: "; sub = pickSubarray( A, 3, 9); displayArr( sub ); }
Output
Given Array: 57, 10, 14, 19, 86, 52, 32, 14, 76, 65, 32, 14, Sub array from index 3 to 9: 19, 86, 52, 32, 14, 76,
Conclusion
Here we have seen three different approaches to creating a sublist from a given array. From the array and two indices i and j ( assume the start and end indices), another new array is being formed. In the first approach, we are defining a blank array first, then copy the elements one by one from the start index to the end index. The second and third approaches are based on vectors which are dynamic arrays present in C++ STL. The second solution is showing a kind of manual (user−defined) method for copying elements from one vector to the final vector. Manually picking elements using for loop. And in the last approach, we are using STL based approach for a faster and cleaner solution. In that solution, the iterators or pointers are used to directly pick elements from the starting index to the ending index.