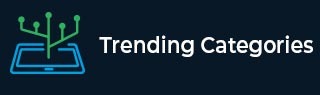
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program to get the imaginary part of the given complex number
Modern science relies heavily on the concept of complex numbers, which was initially established in the early 17th century. The formula for a complex number is a + ib, where a and b are real values. A complex number is known to have two parts: the real component (a) and the imaginary part (ib). The i or iota has the value √-1. The complex class in C++ is a class that is used to represent complex numbers. The complex class in C++ can represent and control several complex number operations. We take a look at how to represent and display complex numbers.
The imag() member function
As discussed earlier, a complex number has two parts, the real part, and the imaginary part. To display the real part we use the real() function and the imag() function is used to display the imaginary part of a given complex number. In the next example, we take a complex number object, initialize it and then display the real and imaginary parts of the number separately.
Syntax
//displaying the imaginary part only complex<double> c; cout << imag(c) << endl;
Algorithm
Take a new complex number object.
Assign a value to the object using the ‘a. + ib’ notation.
Display the imaginary part of the complex number value using the imag() function.
Example
#include <iostream> #include <complex> using namespace std; //displays the imaginary part of the complex number void display(complex <double> c){ cout << "The imaginary part of the complex number is: "; cout << imag(c) << endl; } //initializing the complex number complex<double> solve( double real, double img ){ complex<double> cno(real, img); return cno; } int main(){ complex<double> cno = 10.0 + 11i; //displaying the complex number cout << "The complex number is: " << real(cno) << '+' << imag(cno) << 'i' << endl; display(cno); return 0; }
Output
The complex number is: 10+11i The imaginary part of the complex number is: 11
One thing has to be noted the imag() function takes only complex numbers as input and returns the imaginary part of the provided complex number. The output value is the template parameter of the complex number object.
Conclusion
For many distinct operations throughout a wide spectrum of scientific disciplines, complex numbers are required. An interface for representing complex numbers is provided by the C++ complex class, which is part of the header <complex>. All complex number operations, including addition, subtraction, multiplication, conjugation, norm, and a great deal more, are supported by the complex class. Complex numbers can easily be created using conventional numerical values, as we've just covered in this article. It is important to keep in mind that when presenting complex numbers, the real and imaginary components of the number must both be taken into consideration. Otherwise, several issues could arise. The programmer has to make sure that they do not make mistakes between the real() and the imag() function or otherwise, the values will be reversed.