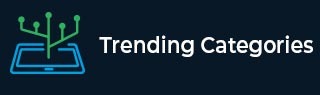
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program to find the hyperbolic arccosine of the given value
Similar to regular trigonometric functions, hyperbolic functions are defined using the hyperbola rather than the circle. From the specified radian angle, it returns the ratio parameter in the hyperbolic cosine function. But, to put it another way, to do the opposite. Inverse hyperbolic trigonometric operations like the hyperbolic arccosine operation are needed to determine the angle from the hyperbolic-cosine value.
To calculate the angle using the hyperbolic cosine value, in radians, this tutorial will show how to use the C++ hyperbolic inverse-cosine (acosh) function. The formula for the hyperbolic inverse-cosine operation is as follows −
$$\mathrm{cosh^{-1}x\:=\:In(x\:+\:\sqrt{x^2\:-\:1})},where \:In\: is\: natural\: logarithm\:(log_e \: k)$$
The acosh() function
Using the acosh() function, the angle may be determined from the hyperbolic cosine value. The C++ standard library includes this function. Before using this function, the cmath library must be imported. This method accepts a hyperbolic cosine value as an input and returns the angle in radians. Simple syntax is used in the following −
Syntax
#include < cmath > acosh( <hyperbolic cosine value> )
The input range for this function is 1 and above. If the input is negative, it will raise domain error. It returns a number in range [0, +∞] (both included).
Algorithm
- Take hyperbolic cosine value x as input
- Use acosh( x ) to calculate the cosh−1(x)
- Return result.
Example
#include <iostream> #include <cmath> using namespace std; float solve( float x ) { float answer; answer = acosh( x ); return answer; } int main() { float angle, ang_deg; angle = solve( 2.50918 ); ang_deg = angle * 180 / 3.14159; cout << "The angle (in radian) for given hyperbolic cosine value 2.50918 is: " << angle << " = " << ang_deg << " (in degrees)" << endl; angle = solve( 11.5919 ); ang_deg = angle * 180 / 3.14159; cout << "The angle (in radian) for given hyperbolic cosine value 11.5919 is: " << angle << " = " << ang_deg << " (in degrees)" << endl; angle = solve( 1.32461 ); ang_deg = angle * 180 / 3.14159; cout << "The angle (in radian) for given hyperbolic cosine value 1.32461 is: " << angle << " = " << ang_deg << " (in degrees)" << endl; angle = solve( 1.60028 ); ang_deg = angle * 180 / 3.14159; cout << "The angle (in radian) for given hyperbolic cosine value 1.60028 is: " << angle << " = " << ang_deg << " (in degrees)" << endl; }
Output
The angle (in radian) for given hyperbolic cosine value 2.50918 is: 1.5708 = 90.0001 (in degrees) The angle (in radian) for given hyperbolic cosine value 11.5919 is: 3.14159 = 180 (in degrees) The angle (in radian) for given hyperbolic cosine value 1.32461 is: 0.785399 = 45.0001 (in degrees) The angle (in radian) for given hyperbolic cosine value 1.60028 is: 1.04719 = 59.9997 (in degrees)
The hyperbolic cosine value is passed to the acosh() method, which returns the angle in radian format. Using the algorithm below, we changed this output from radians to degrees.
$$\mathrm{\theta_{deg}\:=\:\theta_{rad}\:\times\frac{180}{\pi}}$$
Conclusion
We employ the acosh() function from the cmath package to perform the inverse hyperbolic operation using the hyperbolic cosine value. This function outputs the desired angle in radians from the input value of the hyperbolic cosine. The range of the return is 0 to positive infinity. The domain error is raised when the input value is less than 1. The return type in earlier iterations of C and C++ was double; later iterations of C++ also used the overloaded form for float and long-double. When an integer value is supplied as an argument, the acosh() function will be called after casting the input parameter into the double type.