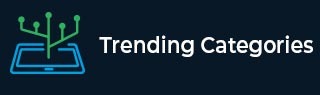
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ program to count final number of characters are there after typing n characters in a crazy writer
Suppose we have an array A with n elements, and another value c is there. There is a crazy word processor present in our system where we can type characters but if we do not type for consecutive c seconds, all of the written letters will be removed. A[i] represents the time when we have typed the ith character. We have to find the final number of characters that will remain on the screen after typing all n characters.
So, if the input is like A = [1, 3, 8, 14, 19, 20]; c = 5, then the output will be 3, because at the second 8 there will be 3 words on the screen. Then, everything disappears at the second 13. At the seconds 14 and 19 another two characters are typed, and finally, at the second 20, one more character is typed, and a total of 3 letters remain on the screen.
Steps
To solve this, we will follow these steps −
s := 1 n := size of A for initialize i := 1, when i < n, update (increase i by 1), do: if (A[i] - A[i - 1]) <= c, then: (increase s by 1) Otherwise s := 1 return s
Example
Let us see the following implementation to get better understanding −
#include <bits/stdc++.h> using namespace std; int solve(vector<int> A, int c) { int s = 1; int n = A.size(); for (int i = 1; i < n; i++) { if ((A[i] - A[i - 1]) <= c) { s++; } else { s = 1; } } return s; } int main() { vector<int> A = { 1, 3, 8, 14, 19, 20 }; int c = 5; cout << solve(A, c) << endl; }
Input
{ 1, 3, 8, 14, 19, 20 }, 5
Output
3