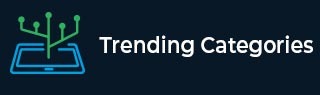
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program string class and its applications?
String is a sequence of characters. In C++ programming language, The strings can be defined in two ways −
C style strings: treats the string as a character array.
String Class in C++
The string class can be used in a C++ program from the library ‘string’. It stores the string as a character array in the memory but shows it as a string object to the user. In C++ there are many methods that support the C++ string class and help in the proper function of the object and increase the efficiency of the code.
Example
Some common string application are found where string are used −
#include <bits/stdc++.h> using namespace std; bool charcheck(string str) { int l = str.length(); for (int i = 0; i < l; i++) { if (str.at(i) < '0' || str.at(i) > '9') return false; } return true; } string replacedotWith20(string str) { string replaceby = "%20"; int n = 0; while ((n = str.find(" ", n)) != string::npos ) { str.replace(n, 1, replaceby); n += replaceby.length(); } return str; } int main() { string num = "3452i"; if (charcheck(num)) cout << "string contains only digit" << endl; else cout<<"string contains other characters too"<<endl; string url = "google com in"; cout << replacedotWith20(url) << endl; return 0; }
Output
String contains other characters too.
google%20com%20in
The above thing can be help while working with web.
Advertisements