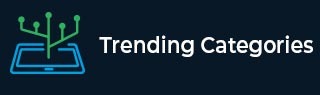
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program for Recursive Bubble Sort?
In this section we will see another approach of famous bubble sort technique. We have used bubble sort in iterative manner. But here we will see recursive approach of the bubble sort. The recursive bubble sort algorithm is look like this.
Algorithm
bubbleRec(arr, n)
begin if n = 1, return for i in range 1 to n-2, do if arr[i] > arr[i+1], then exchange arr[i] and arr[i+1] end if done bubbleRec(arr, n-1) end
Example
#include<iostream> using namespace std; void recBubble(int arr[], int n){ if (n == 1) return; for (int i=0; i<n-1; i++) //for each pass p if (arr[i] > arr[i+1]) //if the current element is greater than next one swap(arr[i], arr[i+1]); //swap elements recBubble(arr, n-1); } main() { int data[] = {54, 74, 98, 154, 98, 32, 20, 13, 35, 40}; int n = sizeof(data)/sizeof(data[0]); cout << "Sorted Sequence "; recBubble(data, n); for(int i = 0; i <n;i++){ cout << data[i] << " "; } }
Output
Sorted Sequence 13 20 32 35 40 54 74 98 98 154
Advertisements