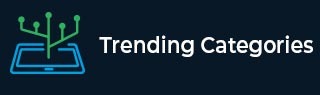
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program for GCD 0.of more than two (or array) numbers?
Here we will see how we can get the gcd of more than two numbers. Finding gcd of two numbers are easy. When we want to find gcd of more than two numbers, we have to follow the associativity rule of gcd. For example, if we want to find gcd of {w, x, y, z}, then it will be {gcd(w,x), y, z}, then {gcd(gcd(w,x), y), z}, and finally {gcd(gcd(gcd(w,x), y), z)}. Using array it can be done very easily.
Algorithm
gcd(a, b)
begin if a is 0, then return b end if return gcd(b mod a, a) end
getArrayGcd(arr, n)
begin res := arr[0] for i in range 1 to n-1, do res := gcd(arr[i], res) done return res; end
Example
#include<iostream> using namespace std; int gcd(int a, int b) { if (a == 0) return b; return gcd(b%a, a); } int getArrayGcd(int arr[], int n) { int res = arr[0]; for(int i = 1; i < n; i++) { res = gcd(arr[i], res); } return res; } main() { int arr[] = {4, 8, 16, 24}; int n = sizeof(arr)/sizeof(arr[0]); cout << "GCD of array elements: " << getArrayGcd(arr, n); }
Output
GCD of array elements: 4
Advertisements