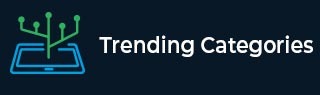
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program for Coefficient of variation
We are given with an array of float values of size n and the task is to find the coefficient of variation and display the result.
What is the coefficient of variation?
In statistic measure, coefficient of variation is used to find the range of variability through the data given. In terms of finance, coefficient of variation is used to find the amount of risk involved with respect to the amount invested. If the ratio between standard deviation and mean is low then the risk involved in the investment is also low. Coefficient of variation is the ratio between standard deviation and mean and it is given by −
Coefficient of variation = Standard Deviation / Mean
Example
Input-: array[] = { 10.0, 21, 23, 90.0, 10.5, 32.56, 24, 45, 70.0 } Output-: coefficient of variation is : 0.75772 Input-: array[] = { 15.0, 36.0, 53.67, 25.45, 67.8, 56, 78.09} Output-: coefficient of variation is : 0.48177
Approach used in the given program is as follows −
- Input the array containing float values
- Calculate the value of mean and standard deviation on the given array
- Calculate the value of coefficient of variation by dividing the value of standard deviation with mean
- Display the result as coefficient of variation
Algorithm
Start Step 1-> declare function to calculate the value of mean float cal_mean(float arr[], int size) Declare float sum = 0 Loop For i = 0 and i < size and i++ Set sum = sum + arr[i] End return sum / size Step 2-> declare function to calculate the value of standard deviation float StandardDeviation(float arr[], int size) Declare float sum = 0 Loop For i = 0 and i < size and i++ Set sum = sum + (arr[i] - cal_mean(arr, size)) * (arr[i] - End Call cal_mean(arr, size)) return sqrt(sum / (size - 1)) Step 3-> Declare function to calculate coefficient of variation float CoefficientOfVariation(float arr[], int size) return StandardDeviation(arr, size) / cal_mean(arr, size) Step 4-> In main() Declare an array of float arr[] = { 10.0, 21, 23, 90.0, 10.5, 32.56, 24, 45, 70.0} Calculate the size of array as int size = sizeof(arr) / sizeof(arr[0]) Call function as CoefficientOfVariation(arr, size) Stop
Example
#include <bits/stdc++.h> using namespace std; // function to calculate the mean. float cal_mean(float arr[], int size) { float sum = 0; for (int i = 0; i < size; i++) sum = sum + arr[i]; return sum / size; } //function to calculate the standard deviation float StandardDeviation(float arr[], int size) { float sum = 0; for (int i = 0; i < size; i++) sum = sum + (arr[i] - cal_mean(arr, size)) * (arr[i] - cal_mean(arr, size)); return sqrt(sum / (size - 1)); } // function to calculate the coefficient of variation. float CoefficientOfVariation(float arr[], int size) { return StandardDeviation(arr, size) / cal_mean(arr, size); } int main() { float arr[] = { 10.0, 21, 23, 90.0, 10.5, 32.56, 24, 45, 70.0}; int size = sizeof(arr) / sizeof(arr[0]); cout<<"coefficient of variation is : "<<CoefficientOfVariation(arr, size); return 0; }
Output
coefficient of variation is : 0.75772
Advertisements