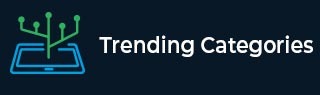
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Program for class interval arithmetic mean
We are given with three arrays where first array contains the upper limit for arithmetic mean, second array contains the lower limit for arithmetic mean and third array contains the frequencies and the task is to generate the arithmetic mean of class intervals given.
What is Arithmetic Mean?
Arithmetic mean is the average value that is calculated by dividing the sum of all the elements in the set with the total number of elements in the given set.
How to calculate Class Interval Arithmetic Mean
- Given with Lower Limit, Upper Limit, Frequency
Lower Limit | Upper Limit | Frequency |
---|---|---|
1 | 2 | 1 |
3 | 4 | 2 |
5 | 6 | 3 |
7 | 8 | 4 |
- Calculate the mid-point by adding the upper limit and lower limit and dividing the final result with 2 since there are two values. After that multiply the mid-point of class interval with its corresponding frequency.
Lower Limit | Upper Limit | Frequency(f) | Mid-Point(m) | m*f |
---|---|---|---|---|
1 | 2 | 1 | (1+2)/2=1.5 | 1.5*1=1.5 |
3 | 4 | 2 | (3+4)/2=3.5 | 3.5*2=7.0 |
5 | 6 | 3 | (5+6)/2=5.5 | 5.5*3=16.5 |
7 | 8 | 4 | (7+8)/2=7.5 | 7.5*4=30.0 |
- Calculate the arithmetic mean by dividing the sum of m*f with the sum of frequencies for final result.
class Interval Arithmetic Mean = sum of m*f/sum of f = (1.5+7.0+16.5+30.0)/(1+2+3+4) = 5.5
Example
Input-: LowerLimit[] = {1, 6, 11, 16, 21} UpperLimit[] = {5, 10, 15, 20, 25} freq[] = {10, 20, 30, 40, 50} Output: 16.3333 Input-: UowerLimit[] = { 2, 4, 6, 8, 10 } LpperLimit[] = { 1, 3, 5, 7, 9 } freq[] = { 1, 2, 3, 4, 5 } Output: 5.5
Algorithm
START Step 1-> declare function to calculate class interval arithmetic mean float AM(int LowerLimit[], int UpperLimit[], int freq[], int terms) Declare float mid[terms] declare and set float sum = 0 and Sum_freq = 0 Loop For int i = 0 and i < terms and i++ Set mid[i] = (LowerLimit[i] + UpperLimit[i]) / 2 Set sum = sum + mid[i] * freq[i] Set Sum_freq = Sum_freq + freq[i] End return sum / Sum_freq Step 2-> In main() Declare int LowerLimit[] = { 2, 4, 6, 8, 10 } Declare int UpperLimit[] = { 1, 3, 5, 7, 9 } Declare int freq[] = { 1, 2, 3, 4, 5 } Declare int size = sizeof(freq) / sizeof(freq[0]) Call AM(LowerLimit, UpperLimit, freq, size) STOP
Example
#include <bits/stdc++.h> using namespace std; //calculate class interval arithmetic mean. float AM(int LowerLimit[], int UpperLimit[], int freq[], int terms) { float mid[terms]; float sum = 0, Sum_freq = 0; for (int i = 0; i < terms; i++) { mid[i] = (LowerLimit[i] + UpperLimit[i]) / 2; sum = sum + mid[i] * freq[i]; Sum_freq = Sum_freq + freq[i]; } return sum / Sum_freq; } int main() { int UowerLimit[] = { 2, 4, 6, 8, 10 }; int LpperLimit[] = { 1, 3, 5, 7, 9 }; int freq[] = { 1, 2, 3, 4, 5 }; int size = sizeof(freq) / sizeof(freq[0]); cout<<"Arithmetic mean is : "<<AM(LowerLimit, UpperLimit, freq, size); return 0; }
Output
Arithmetic mean is : 5.5
Advertisements