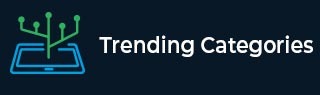
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Partitioning a Linked List Around a Given Value and Keeping the Original Order
Given a linked list in this tutorial, and we need to keep all the numbers smaller than x at the start of the list and the others at the back. We also need to retain their order the same as previously. for example
Input : 1->4->3->2->5->2->3, x = 3 Output: 1->2->2->3->3->4->5 Input : 1->4->2->10 x = 3 Output: 1->2->4->10 Input : 10->4->20->10->3 x = 3 Output: 3->10->4->20->10
To solve this problem, we need to make three linked lists now. When we encounter a number smaller than x, then we insert it into the first list. Now for a value equal to x, we put it in second, and for the greater value, we insert it in third now. In the end, we concatenate the lists and print the final list.
Approach to Find the Solution
In this approach, we are going to maintain three lists, namely small, equal, large. Now we maintain their order and concatenate the lists into a final list, which is our answer.
Example
C++ Code for the Above Approach
#include<bits/stdc++.h> using namespace std; struct Node{ // structure for our node int data; struct Node* next; }; // A utility function to create a new node Node *newNode(int data){ struct Node* new_node = new Node; new_node->data = data; new_node->next = NULL; return new_node; } struct Node *partition(struct Node *head, int x){ struct Node *smallhead = NULL, *smalllast = NULL; // we take two pointers for the list //so that it will help us in concatenation struct Node *largelast = NULL, *largehead = NULL; struct Node *equalhead = NULL, *equallast = NULL; while (head != NULL){ // traversing through the original list if (head->data == x){ // for equal to x if (equalhead == NULL) equalhead = equallast = head; else{ equallast->next = head; equallast = equallast->next; } } else if (head->data < x){ // for smaller than x if (smallhead == NULL) smalllast = smallhead = head; else{ smalllast->next = head; smalllast = head; } } else{ // for larger than x if (largehead == NULL) largelast = largehead = head; else{ largelast->next = head; largelast = head; } } head = head->next; } if (largelast != NULL) // largelast will point to null as it is our last list largelast->next = NULL; /**********Concatenating the lists**********/ if (smallhead == NULL){ if (equalhead == NULL) return largehead; equallast->next = largehead; return equalhead; } if (equalhead == NULL){ smalllast->next = largehead; return smallhead; } smalllast->next = equalhead; equallast->next = largehead; return smallhead; } void printList(struct Node *head){ // function for printing our list struct Node *temp = head; while (temp != NULL){ printf("%d ", temp->data); temp = temp->next; } } int main(){ struct Node* head = newNode(10); head->next = newNode(4); head->next->next = newNode(5); head->next->next->next = newNode(30); head->next->next->next->next = newNode(2); head->next->next->next->next->next = newNode(50); int x = 3; head = partition(head, x); printList(head); return 0; }
Output
2 10 4 5 30
Explanation of the Above Code
In the approach described above, we'll keep three linked lists with the contents in sequential sequence. These three linked lists will contain elements smaller than, equal, and greater than x separately. Our task is simplified now. We need to concatenate the lists and then return the head.
Conclusion
In this tutorial, we solve Partitioning a linked list around a given value and keeping the original order. We also learned the C++ program for this problem and the complete approach (Normal) by which we solved this problem. We can write the same program in other languages such as C, java, python, and other languages. We hope you find this tutorial helpful.