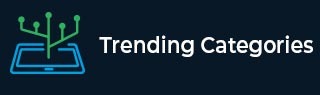
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ code to find answers by vowel checking
Suppose we have a string S. Amal and Bimal are playing a game. The rules of the game are like: Those who play for the first time, that is Amal is the detective, he should investigate a "crime" and find out the cause. He can ask any questions whose answers will be either "Yes" or "No". If the question’s last letter is a vowel, they answer "Yes" otherwise "No". Here the vowels are: A, E, I, O, U, Y. We have S as question and we have to find the answer.
So, if the input is like S = "Is it in university?", then the output will be Yes.
Steps
To solve this, we will follow these steps −
s := "AEIOUYaeiouy" for initialize i := 0, when i < size of S, update (increase i by 1), do: t := S[i] if t is alphabetic, then: ans := t if ans is in s, then: return "YES" Otherwise return "NO"
Example
Let us see the following implementation to get better understanding −
#include <bits/stdc++.h> using namespace std; string solve(string S){ string s = "AEIOUYaeiouy"; char ans; for (int i = 0; i < S.size(); i++){ char t = S[i]; if (isalpha(t)) ans = t; } if (s.find(ans) != -1) return "YES"; else return "NO"; } int main(){ string S = "Is it in university?"; cout << solve(S) << endl; }
Input
"Is it in university?"
Output
YES
Advertisements