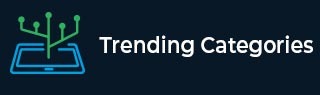
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C++ Balanced expressions such that given positions have opening brackets
A balanced expression of parentheses is an expression that contains pairs of all sort of parentheses together in a correct order. this means that for every opening parentheses there is a closing parentheses in proper order of parentheses i.e. { }.
Expression − {([][]{})({}[]{})}
Output − balanced
Now, in this problem we have to create all possiblebalanced expressions from the given number of brackets.And the condition is that the given position have opening brackets.
In this problem, we are given an integer n and an array of position of the brackets of length 2n and we have to find the number of balanced expressions of length 2n in such a way that the positions marked by opening bracket will have opening bracket ‘{’.
Example −
Input : n = 2 , position [1, 0 , 0 , 0]. Output : 2 Explanation : All possible outcomes are : {{}} , {}{}.
ALGORITHM
All positions with one are open brackets.
Use a recursive loop using the following rules,
If the ( number of opening brackets - number of closing brackets ) > 0 , return 0.
After looping till n and if the total brackets after push and pop is 0, then return 1 i.e.solution obtained. Otherwise return 0.
If 1 is pre-assigned to the expression, call recursively on increasing the index and increase the total number of brackets.
Else call the function recursively by inserting open brackets at index place and then insert the closed bracket for it and decrease the total number of brackets and move to the next index.
PROGRAM
#include <bits/stdc++.h> using namespace std; int find(int index, int openbrk, int n, int expression[]){ if (openbrk < 0) return 0; if (index == n){ if (openbrk == 0) return 1; else return 0; } if (expression[index] == 1) { return find(index + 1, openbrk + 1, n, expression); } else { return find(index + 1, openbrk + 1, n, expression) + find(index + 1, openbrk - 1, n, expression); } } int main() { int n = 3; int expression[6] = { 1, 0, 1, 0, 0, 0}; cout << find(0, 0, 2 * n, expression) <<endl; return 0; }