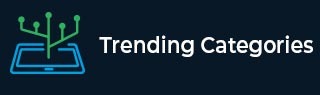
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Counting pairs with range sum in a limit in JavaScript
Range Sum
Range sum rangeSum(i, j) is defined as the sum of the elements in an array between indices i and j (i ≤ j), inclusive.
Problem
We are required to write a JavaScript function that takes in an array of Integers, arr, as the first argument and two numbers, upper and lower as the second and third element.
Our function is supposed to return the number of range sums that lie between the range [upper, lower], (both inclusive).
For example, if the input to the function is −
const arr = [1, 4, 3]; const upper = 5; const lower = 2;
Then the output should be −
const output = 3;
Example
The code for this will be −
const arr = [1, 4, 3]; const upper = 5; const lower = 2; const countRangeSum = (arr = [], lower, upper) => { const sums = [0]; let res = 0; let last = 0; let firstge = value => { let l = 0, r = sums.length, m; do { m = Math.floor((r + l) / 2); sums[m] < value ? l = m : r = m; } while (r >= l + 2); while (r > 0 && sums[r - 1] >= value ) { r -= 1; } return r; }; arr.forEach(num => { last += num; res += firstge(last - lower + 1) - firstge(last - upper); sums.splice(firstge(last), 0, last); }); return res; }; console.log(countRangeSum(arr, lower, upper));
Output
The output in the console will be −
3
Advertisements