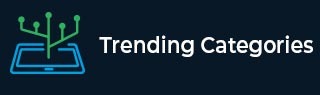
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Counting Number of Dinosaurs in Python
Suppose we have a string called animals and another string called dinosaurs. Every letter in animals represents a different type of animal and every unique character in dinosaurs string represents a different dinosaur. We have to find the total number of dinosaurs in animals.
So, if the input is like animals = "xyxzxyZ" dinosaurs = "yZ", then the output will be 3, as there are two types of dinosaurs y and Z, in the animal string there are two y type animal and one Z type animal.
To solve this, we will follow these steps −
- res := 0
- dinosaurs := a new set by taking elements from dinosaurs
- for each c in dinosaurs, do
- res := res + occurrence of c in animals
- return res
Let us see the following implementation to get better understanding −
Example
class Solution: def solve(self, animals, dinosaurs): res = 0 dinosaurs = set(dinosaurs) for c in dinosaurs: res += animals.count(c) return res ob = Solution() animals = "xyxzxyZ" dinosaurs = "yZ" print(ob.solve(animals, dinosaurs))
Input
"xyxzxyZ", "yZ"
Output
3
Advertisements