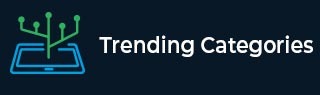
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count ways to spell a number with repeated digits in C++
We are given a number containing many repeated digits as a string. The goal is to find the number of ways to spell it. For example 112233 can be spelled as double one, double two double three or one one two two three three.
We will do this by checking continuous numbers. If the number is “13” there is only one way to spell it as “one three” (20). If digits are “113” ways “double one three”, “one one three” (21). So, the approach is to count the one continuous digit in string and multiply 2(count-1) with the previous result.
Let’s understand with examples.
Input
num=”11211”
Output
Count of ways to spell a number with repeated digits are: 4
Explanation
ways are: 1. One one two one one 2. Double one two one one 3. One one two double one 4. Double one two double one
Input
num=”2212”
Output
Count of ways to spell a number with repeated digits are: 2
Explanation
ways are: 1. Two two one two 2. Double two one two
Approach used in the below program is as follows
We are taking string str to represent a number.
Function word_spell(string str) takes the number in str and returns ways to spell it.
Take the initial variable count as 0 for such ways
Using for loop traverse str for each digit.
Take a variable temp as the number of repetitions of a particular digit. If str[i]==str[i+1], increase temp.
Calculate count=count*pow(2,temp-1)
At the end return count as result.
Example
#include<bits/stdc++.h> using namespace std; long long int word_spell(string str){ long long int count = 1; int len = str.length(); for (int i=0; i<len; i++){ int temp = 1; while(i < len-1 && str[i+1] == str[i]){ temp++; i++; } count = count * pow(2, temp-1); } return count; } int main(){ string str = "222211"; cout<<"Count of ways to spell a number with repeated digits are: "<<word_spell(str); return 0; }
Output
If we run the above code it will generate the following output −
Count of ways to spell a number with repeated digits are: 16