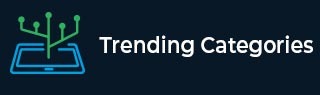
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count the number of non-increasing subarrays in C++
Given an array arr[] containing positive integers. The goal is to find the number of subarrays of length at least 1 which are non−increasing. If arr[]= {1,3,2}, then subarrays will be {1}, {2}, {3}, {3,2}. Count is 4.
For Example
Input
arr[] = {5,4,5}
Output
Count of number of non-increasing subarrays are: 7
Explanation
The subarrays will be − {5}, {4}, {5}, {5,4}
Input
arr[] = {10,9,8,7}
Output
Count of number of non−increasing subarrays are − 10
Explanation
The subarrays will be − {10}, {9}, {8}, {7}, {10,9}, {9,8}, {8,7}, {10,9,8}, {9,8,7}, {10,9,8,7}
Approach used in the below program is as follows −
In this approach, we will use the fact that if elements between indexes i and j in arr[] are not non−increasing then elements between indexes i to j+1, i to j+2….i to j+n−1 can never be non increasing so keep incrementing the length of this subarray till elements are non-increasing. If any lesser element is found arr[j]<arr[j−1] then add len*(len+1)/2 ( subarrays of current non−increasing subarray containing len elements ) to count such subarrays and reset the length of the subarray to 1.
Take an integer array arr[].
Function subarrays(int arr[], int size) takes the array and its size and returns the count of the number of non-increasing subarrays.
Take the initial count as 0 and length of smallest subarray as temp=1.
Traverse arr[] using for loop, if arr[i + 1] <= arr[i] then increment temp as subarray is non−increasing.
Otherwise add (temp + 1) * temp) / 2 to count for number of subrrays of subarray of length temp which is non−increasing.
Set temp=1 for new subarray.
At the end of all loops, if length temp>1 again add (temp + 1) * temp) / 2 to count for last subarray.
Return count as result.
Example
#include <bits/stdc++.h> using namespace std; int subarrays(int arr[], int size){ int count = 0; int temp = 1; for(int i = 0; i < size − 1; ++i){ if (arr[i + 1] <= arr[i]){ temp++; } else { count += (((temp + 1) * temp) / 2); temp = 1; } } if(temp > 1){ count += (((temp + 1) * temp) / 2); } return count; } int main(){ int arr[] = {2, 6, 1, 8, 3}; int size = sizeof(arr) / sizeof(arr[0]); cout<<"Count of number of non−increasing subarrays are: "<<subarrays(arr, size); return 0; }
Output
If we run the above code it will generate the following output −
Count the number of non-increasing subarrays are: 7