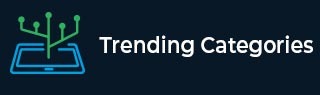
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count pairs of natural numbers with GCD equal to given number in C++
We gave three input variables as ‘start’, ‘end’ and ‘number’. The goal is to find pairs of numbers between start and end that have GCD value equal to ‘number’. For example GCD(A,B)=number and both A, B are in range [start,end].
Let us understand with examples.
Input − start=5 end=20 number=8
Output − Count of pairs of natural numbers with GCD equal to given number are − 3
Explanation − Pairs between 5 to 20 such that GCD is 8 are − (8,8), (8,16), (16,8)
Input − start=5 end=20 number=7
Output − Count of pairs of natural numbers with GCD equal to given number are − 2
Explanation− Pairs between 20 to 30 such that GCD is 7 are − (21,28), (28,21)
Approach used in the below program is as follows
We will use two approaches. First naive approach in which we will traverse using for loop from i=start to i<=end and inner loop from j=start to j<=end. For each pair(i,j) check if GCD(i,j)==number. If true increment count.
Take the variables start, end and number as integers.
Function GCD(int a, int b) is recursive and returns the GCD of arguments a,b passed to it.
If b is non-zero it calls itself recursively as GCD(b,a%b) else it returns a.
Function GCD_pairs(int start, int end, int number) takes boundary variables start, end and variable number and returns pairs between start and end that have gcd=number.
Take the initial count as 0.
Using two for loops for each member of the pair. Outer loop from i=start to i<=end and inner loop from j=start to j<=end.
Check if for pair (i,j), GCD(i,j)==number. If true, increment count.
At last we will get the total count of pairs with gcd=number.
Return count as result.
Efficient Approach
In this approach, we will update the values of start and end. For pair(i,j) to have gcd=number, both i,j should be divisible by ‘number. There will be at-most (end-start)/number no.s that will fully divide ‘number’. For getting numbers between start and end that are divisible by ‘number’, we will traverse from start = (start + number - 1) / number to end = end / number; So for each such numbers if gcd(i,j)==1 then increment count for such pair (i,j).
Take the variables start, end and number as integers.
Update start = (start+number - 1)/number. And end=end/number.
Function GCD(int a, int b) is recursive and returns the GCD of arguments a,b passed to it.
If b is non-zero it calls itself recursively as GCD(b,a%b) else it returns a.
Function GCD_pairs(int start, int end, int number) takes boundary variables start, end and variable number and returns pairs between start and end that have gcd=number.
Take the initial count as 0.
Using two for loops for each member of the pair. Outer loop from i=start to i<=end and inner loop from j=start to j<=end.
Check if for pair (i,j), GCD(i,j)==1. If true, increment count.
At last we will get the total count of pairs with gcd=number.
Return count as result.
Example (naive approach)
#include <bits/stdc++.h> using namespace std; int GCD(int a, int b){ return b ? GCD(b, a % b) : a; } int GCD_pairs(int start, int end, int number){ int count = 0; for (int i = start; i <= end; i++){ for (int j = start; j <= end; j++){ if (GCD(i, j) == number){ count++; } } } return count; } int main(){ int start = 10, end = 30, number = 10; cout<<"Count of pairs of natural numbers with GCD equal to given number are: "<<GCD_pairs(start, end, number) << endl; return 0; }
Output
If we run the above code it will generate the following output −
Count of pairs of natural numbers with GCD equal to given number are: 7
Example (Efficient approach)
#include <bits/stdc++.h> using namespace std; int GCD(int a, int b){ return b ? GCD(b, a % b) : a; } int GCD_pairs(int start, int end, int number){ int count = 0; for (int i = start; i <= end; i++){ for (int j = start; j <= end; j++){ if (GCD(i, j) == 1){ count++; } } } return count; } int main(){ int start = 10, end = 30, number = 10; start = (start + number - 1) / number; end = end / number; cout<<"Count of pairs of natural numbers with GCD equal to given number are: "<<GCD_pairs(start, end, number) << endl; return 0; }
Output
If we run the above code it will generate the following output −
Count of pairs of natural numbers with GCD equal to given number are: 7