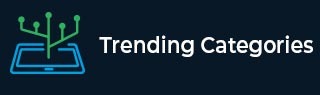
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count pairs from two arrays having sum equal to K in C++
We are given two arrays Arr1[] and Arr2[] and a number K. The goal is to find unique pairs of elements of both arrays such that their sum is K. Pairs will be of form ( Arr1[i], Arr2[j] ) where Arr1[i]+Arr2[j]==K.
We will traverse using two loops for i and j. If sum (Arr1[i]+Arr2[j])==K. And the pair doesn’t exist in unordered_map<int,int>. Add it to the map and increment count.
Let’s understand with examples.
Input
Arr1[]={ 1,3,2,4,3,2 }; Arr2[]={ 0,2,1,2,3 }; K=4
Output
Number of pairs with sum K : 4
Explanation
Pairs will be ( Arr1[0], Arr2[4] ) → (1,3) ( Arr1[1], Arr2[2] ) → (3,1) ( Arr1[2], Arr2[1] ) → (2,2) ( Arr1[3], Arr2[2] ) → (3,1) All other pairs already exist.Total unique pairs 4.
Input
Arr1[]={ 0,2,1,2,3}; Arr2[]={ 1,1,1,1,1 }; K=3
Output
Number of pairs with sum K : 1
Explanation
Pairs will be ( Arr1[1], Arr2[0] ) → (2,1) All other pairs already exist.Total unique pairs 1.
Approach used in the below program is as follows
We take two arrays Arr1[] and Arr2[] and a variable K for sum.
Len1 and Len2 are used to denote the length of both arrays.
Function pairsumisK(int arr1[],int arr2[],int k,int l1,int l2) takes all variables and returns the count of unique pairs of elements from both arrays with sum=k.
Take the initial variable count as 0 for pairs.
Take unordered_map umap to store unique pairs.
Traverse both arrays using two for loops.
For elements in arr1[] from i=0 to i<len1. For elements in arr2[] from j=0 to j<len2.
Check if sum arr1[i]+arr2[j]=k. If yes, check if this pair exists through umap.find(....)==umap.end().
If it doesn’t add this pair to umap and increment count.
At the end of all loops count will have a total number of such pairs.
Return the count as result.
Example
#include <bits/stdc++.h> using namespace std; int pairsumisK(int arr1[],int arr2[],int k,int l1,int l2){ int count = 0; unordered_map<int, int> umap; for (int i = 0; i < l1; i++){ for (int j = 0; j < l2; j++){ int sum=arr1[i]+arr2[j]; if(sum==k) //pairs with sum=k only{ if(umap.find(arr1[i]) == umap.end()) //unique pairs only{ umap.insert(make_pair(arr1[i],arr2[j])); } } } } return count; } int main(){ int Arr1[]={ 1,2,3,0,2,4 }; int Arr2[]={ 3,2,5,2 }; int len1=sizeof(Arr1)/sizeof(Arr1[0]); int len2=sizeof(Arr2)/sizeof(Arr2[0]); int K=5; //length of array cout <<endl<< "Number of pairs with sum K : "<<pairsumisK(Arr1,Arr2,K,len1,len2); return 0; }
Output
If we run the above code it will generate the following output −
Number of pairs with sum K : 0