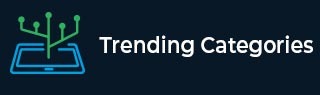
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count ordered pairs with product less than N in C++
We are given a number N. The goal is to find ordered pairs of positive numbers such that their product is less than N.
We will do this by starting from i=1 to i<N and j=1 to (i*j)<N. Then increment count.
Let’s understand with examples.
Input
N=4
Output
Ordered pairs such that product is less than N:5
Explanation
Pairs will be (1,1) (1,2) (1,3) (2,1) (3,1)
Input
N=100
Output
Ordered pairs such that product is less than N: 473
Explanation
Pairs will be (1,1) (1,2) (1,3)....(97,1), (98,1), (99,1). Total 473.
Approach used in the below program is as follows
We take integer N.
Function productN(int n) takes n and returns the count of ordered pairs with product < n
Take the initial variable count as 0 for pairs.
Traverse using two for loops for making pairs.
Start from i=1 to i<n. And j=1 to (i* j)<n.
Increment count by 1.
At the end of all loops count will have a total number of such pairs.
Return the count as result.
Example
#include <bits/stdc++.h> using namespace std; int productN(int n){ int count = 0; for (int i = 1; i < n; i++){ for(int j = 1; (i*j) < n; j++) { count++; } } return count; } int main(){ int N = 6; cout <<"Ordered pairs such that product is less than N:"<<productN(N); return 0; }
Output
If we run the above code it will generate the following output −
Ordered pairs such that product is less than N:10
Advertisements