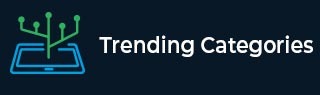
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count of divisors having more set bits than quotient on dividing N in C++
We are given with an integer number let's say, N which is considered as a divisor and it will be divided with the numbers starting from the 1 - N and the task is to calculate the count of those divisors which have more number of set bits than the quotient when divided with the given number N.
For Example
Input - int N = 6
Output - Count of divisors having more set bits than quotient on dividing N are: 5
Explanation - Firstly, we will divide the number N with the numbers starting from 1 - N and calculate the set bits of divisor and the quotient i.e.
1-> N = 6 /1(1) = 6(2) = 1 < 2 = not considered
2-> N = 6 /2(1) = 3(2) = 2 = 2 = considered
3-> N = 6 /3(2) = 2(1) = 2 > 1 = considered
4-> N = 6 /4(1) = 1(1) = 1 = 1 = considered
5-> N = 6 /5(2) = 1(1) = 2 > 1 = considered
6-> N = 6 /6(2) = 1(1) = 2 >1 = considered
As we can see, we will take the considered cases and the output will be 5.
Input - int N = 10
Output - Count of divisors having more set bits than quotient on dividing N are: 8
Explanation - Firstly, we will divide the number N with the numbers starting from 1 - N and calculate the set bits of divisor and the quotient i.e.
1-> N = 10 /1(1) = 10(2) = 1 < 2 = not considered
2-> N = 10 /2(1) = 5(2) = 2 = 2 = considered
3-> N = 10 /3(2) = 3(2) = 2 = 2 = considered
4-> N = 10 /4(1) = 2(1) = 1 < 2 = not considered
5-> N = 10 /5(2) = 2(1) = 2 > 2 = considered
6-> N = 10 /6(2) = 1(1) = 2 >1 = considered
7-> N = 10 /7(3) = 1(1) = 3 >1 = considered
8-> N = 10 /8(1) = 1(1) = 1 = 1 = considered
9-> N = 10 /9(2) = 1(1) = 2 > 2 = considered
10-> N = 10 /10(2) = 1(1) = 2 > 1 = considered
As we can see, we will take the considered cases and the output will be 8.
Approach used in the below program is as follows
- Input a positive integer number N and pass it to the function divisors_quotient() as an argument.
- Inside the function divisors_quotient()
- Return the N - call to the function set_quo(N) + 1 and goto the function set_quo()
- Inside the function set_quo()
- Create a temporary variable as start and end and initialize the start with 1 and end with sqrt(N).
- Start loop WHILE till start < end and create a temporary variable as temp and set it to (start + end) / 2
- Check IF(call to the function verify() and pass temp and N as an argument) then set end as temp
- ELSE, set start as temp + 1
- IF(!call to the function verify() and pass temp and N as an argument) then return start + 1.
- ELSE, return start
- Inside the function verify()
- Check IF(call to the function val_bit(temp/val) is less than call to the function val_bit(val)) then return true otherwise return False
- Inside the function val_bit()
- Declare a temporary variable count to store the result.
- Start loop WHILE val has value. Inside the loop, set val as val / 2 and increase the count by 1.
- Return count.
Example
#include <bits/stdc++.h> using namespace std; int val_bit(int val) { int count = 0; while (val) { val = val / 2; count++; } return count; } bool verify(int val, int temp) { if (val_bit(temp / val) <= val_bit(val)) { return true; } return false; } int set_quo(int N) { int start = 1; int end = sqrt(N); while (start < end) { int temp = (start + end) / 2; if (verify(temp, N)) { end = temp; } else { start = temp + 1; } } if (!verify(start, N)) { return start + 1; } else { return start; } } int divisors_quotient(int N) { return N - set_quo(N) + 1; } int main() { int N = 10; cout << "Count of divisors having more set bits than quotient on dividing N are: " << divisors_quotient(N); return 0; }
If we run the above code it will generate the following output −
Output
Count of divisors having more set bits than quotient on dividing N are: 8