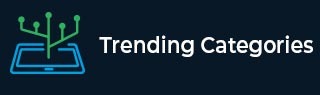
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Count of cells in a matrix which give a Fibonacci number when the count of adjacent cells is added in C++
Given a matrix [ ][ ] having dimensions as row x col. The goal is to find the count of cells of matrix that meet the given condition:
Value of cell matrix [i][j] + no. of adjacent cells to it = a Fibonacci number
Numbers in Fibonacci series:- 0, 1, 1, 2, 3, 5, 8, 13, 21, 43 …..
Let us understand with examples.
For Example
Input - matrix[row][col] = {{1, 4, 1}, {2, 0, 1}, {5, 1, 1}
Output - Count of cells in a matrix which give a Fibonacci number when the count of adjacent cells is added are: 4
Explanation
0 1 2
0 1 4 1
1 2 0 1
2 5 1 1
Cell(0,0) → 1+2=3 ( 2 adjacent cells (1,0) and (0,1) )
Cell(0,2) → 1+2=3
Cell(1,0) → 2+3=5
Cell(2,2) → 1+2=3
Input - matrix[row][col] = {{0,0,0}, {0, 1, 0}, {0, 0, 0} }
Output -Count of cells in a matrix which give a Fibonacci number when the count of adjacent cells is added are: 9
Explanation
0 1 2
0 0 0 0
1 0 1 0
2 0 0 0
Cell(0,0) → 0+2=2 ( 2 adjacent cells (1,0) and (0,1) ) Similarly cells (0,2), (2,2) and (2,0)
Cell(0,1) → 0+3=3 ( 3 adjacent cells (0,1) and (0,2) and (1,1) ) Similarly cells (1,0), (1,2) and (2,1)
Cell (1,1) → 1+4=5
All 9 cells are counted.
Approach used in the below program is as follows
In the matrix of any kind there will be three types of cells only. The corner cells will have 2 adjacent cells, the cells with 3 adjacent cells and the cells with 4 adjacent cells only. Add 2, 3 or 4 to the value of these cells and check if the sum is a fibonacci number using the function check_fibonacci(int num).
- Take a matrix[][] and initialize it.
- Function check_square(long double num) takes a number and returns true if it is a perfect square.
- Function check_fibonacci(int num) returns true if num is a Fibonacci number.
- If check_square(5 * num * num + 4) || check_square(5 * num * num - 4) returns true then num is a Fibonacci number.
- Function Fibonacci_cells(int matrix[row][col]) returns the count of cells in a matrix which gives a Fibonacci number when the count of adjacent cells is added.
- Take the initial count as 0.
- Traverse using for loops from i=0 to i<row and j=0 to j<col. Take total = matrix[i][j].
- Add 2 ,3 or 4 to total based on the cells adjacent to it.
- If the new total is a fibonacci number then check_fibonacci(total) wil return true, so increment count.
- At the end of all for loops return count as result.
Example
#include <bits/stdc++.h> using namespace std; #define row 3 #define col 3 bool check_square(long double num) { long double val = sqrt(num); return ((val - floor(val)) == 0); } bool check_fibonacci(int num) { return check_square(5 * num * num + 4) || check_square(5 * num * num - 4); } int Fibonacci_cells(int matrix[row][col]) { int count = 0; for (int i = 0; i < row; i++) { for (int j = 0; j < col; j++) { int total = matrix[i][j]; if ((i == 0 && j == 0) || (i == row - 1 && j == 0) || (i == 0 && j == col - 1) || (i == row - 1 && j == col - 1)) { total = total + 2; } else if (i == 0 || j == 0 || i == row - 1 || j == col - 1) { total = total + 3; } else { total = total + 4; } if (check_fibonacci(total)) { count++; } } } return count; } int main() { int matrix[row][col] = {{1, 4,1},{2,0,1},{5,1,1}}; cout << "Count of cells in a matrix which give a Fibonacci number when the count of adjacent cells is added are: " << Fibonacci_cells(matrix); return 0; }
If we run the above code it will generate the following output −
Output
Count of cells in a matrix which give a Fibonacci number when the count of adjacent cells is added are: